Add (conditional) debugger breakpoints to your code
In most programming language and most programming environments when you would like to debug some code, you can use an external tool, a debugger, to try to locate problems in your code. This debugger is usually part of an IDE.
With Perl you could use the "build-in" debugger that comes with Perl via the -d flag. See for example how to debug Perl scripts
These external debuggers, including the one that comes with perl allow you to set breakpoints in the code where the execution will stop so you can look around the code and the values of the variables.
Perl allows you to save these breakpoints in your code using the $DB::single variable.
Inserting conditional breakpoint in a complex application
This isn't a really good example because Fibonacci is too simple a case, but imagine that you have a complex and long running application that you'd like to debug.
If you insert the following statement in your code, then when your code is executed using th e built in debugger, it will automatically stop right after executing that assignment.
$DB::single = 1;
Actually you can do even more interesting thing, you can make that assignment conditional, for example like this:
$DB::single = 1 if $n == 6;
That will allow to stop the execution of a function only on certain parameters, or the execution of a loop when you have had a certain number of iterations.
In a simple Fibonacci function it could look like this.
examples/fibonacci_with_breakpoint.pl
use 5.010; use strict; use warnings; no warnings 'once'; my $num = shift or die "Usage: $0 N\n"; say fibonacci($num); sub fibonacci { my ($n) = @_; $DB::single = 1 if $n == 6; return 1 if $n == 1 or $n == 2; return fibonacci($n-1) + fibonacci($n-2); }
You can run this code with any paraamter and that special assignment will have no effect.
perl fibonacci_with_breakpoint.pl 3 2 perl fibonacci_with_breakpoint.pl 7 13
Use the debugger
perl -d fibonacci_with_breakpoint.pl 3
examples/fibonacci_with_breakpoint_1.txt
Loading DB routines from perl5db.pl version 1.53 Editor support available. Enter h or 'h h' for help, or 'man perldebug' for more help. main::(fibonacci_with_breakpoint.pl:6): 6: my $num = shift or die "Usage: $0 N\n"; DB<1>
You type in c at this prompt to tell the debugger to "continue" to the first breakpoint.
As the condition of the breakpoint setting is never met the code will run till the end printing the result and then another prompt:
examples/fibonacci_with_breakpoint_2.txt
2 Debugged program terminated. Use q to quit or R to restart, use o inhibit_exit to avoid stopping after program termination, h q, h R or h o to get additional info. DB<1>
Here you can type q to quit the debugger.
In the second attempt we run the code with a larger input number:
perl -d fibonacci_with_breakpoint.pl 7
We get the first out put and the prompt:
examples/fibonacci_with_breakpoint_1.txt
Loading DB routines from perl5db.pl version 1.53 Editor support available. Enter h or 'h h' for help, or 'man perldebug' for more help. main::(fibonacci_with_breakpoint.pl:6): 6: my $num = shift or die "Usage: $0 N\n"; DB<1>
If we type c here it will continue to run till it encounters the conditional breakpoint and show this:
examples/fibonacci_with_breakpoint_3.txt
main::fibonacci(fibonacci_with_breakpoint.pl:14): 14: return 1 if $n == 1 or $n == 2; DB<1>
At this point we can start using all the other commands of the debugger to look around and explore the situation.
BTW if you are curious what is that no warnings statement, it tells perl to avoid printing the Name "DB::single" used only once: possible typo at warning.
See the full list of warnings.
See also how to debug compile-time code.
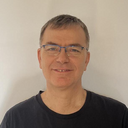
Published on 2019-05-06