bless
The bless function in Perl is used to associate a reference (usually a reference to a hash) with a package to create an instance.
The constructor
The de-facto standard name for the constructor of a class in Perl is the new method. When called with the arrow-notation it will receive the name of the class as the first parameter. (We don't want to assume that the name passed there is the name of our package to allow for inheritance.)
Inside the constructor we create a reference to a hash, then we call bless and pass the reference to this hash and the name of the class. This operation will associate the reference with the given package name. It will also return the blessed reference, but it will also change the argument we passed in.
examples/MyFruit.pm
package MyFruit; use strict; use warnings; sub new { my ($class) = @_; print "Inside new for $class\n"; my $self = {}; print "Self is still only a reference $self\n"; bless $self, $class; print "Self is now associated with the class: $self\n"; return $self; } 1;
Of course you don't need that much code to create the instance, I only wanted to show each step separately. You'll often encounter code like this with implicit return:
sub new { my ($class) = @_; bless {}, $class; }
The usage
The way we can then use the class looks like this:
examples/use_my_fruit.pl
use strict; use warnings; use MyFruit; my $fruit = MyFruit->new; print "The instance: $fruit\n";
We run the code (we need to add the -I. to ensure perl will find the module in the current directory)
perl -I. use_my_fruit.pl
The output will look like this:
Inside new for MyFruit Self is still only a reference HASH(0x55d920554470) Self is now associated with the class: MyFruit=HASH(0x55d920554470) The instance: MyFruit=HASH(0x55d920554470)
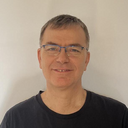
Published on 2021-03-17