Counter using Dancer2 and Redis in a Docker container
In this part of the counter examples series we have Perl Dancer based application using Redis as the in-memory cache/database to store the counter.
The code runs in a Docker container and we have another container running the Redis server.
For this example to work we need the 4 files that you can see below (app.psgi, cpanfile, Dockerfile, docker-compose.yml) in a folder and you have to be in that forlder. You can download the individual files or you can clone the repository of the Perl Maven web site:
git clone https://github.com/szabgab/perlmaven.com.git cd perlmaven.com/examples/redis_counter/
Install Docker and docker-compose then run the command:
docker-compose up
Then you can visit http://localhost:5001 and keep reloading it to see the counter increase.
Ctrl-C will stop the Docker compose.
The Dancer code
examples/redis_counter/app.psgi
use strict; use warnings; use Dancer2; use Redis; my $redis = Redis->new(server => 'myredis:6379'); get '/' => sub { my $counter = $redis->incr('counter'); return $counter; }; dance;
The name of the server "myredis" was defined in the docker-compose.yml file. See below.
cpanfile listing Perl dependencies
examples/redis_counter/cpanfile
requires 'Dancer2', '0.3'; requires 'Redis', '1.998';
Dockerfile for perl and the modules needed
examples/redis_counter/Dockerfile
FROM perl:5.32 WORKDIR /opt COPY cpanfile /opt/ RUN cpanm --installdeps --notest . CMD ["plackup", "--host", "0.0.0.0", "--port", "5000"]
We install the modules using --notest so the installation will be faster. In a real, non-demo application I'd not do that. I'd want the tests to run to verify that the modules I install work properly on my installation.
docker-compose file to bind them all together
examples/redis_counter/docker-compose.yml
version: '3.8' services: web: build: . ports: - "5001:5000" volumes: - .:/opt links: - myredis myredis: image: redis:6.2.1 volumes: - redis-data:/var/lib/redis volumes: redis-data:
Disk usage
The volume storing the redis data:
docker volume inspect redis_counter_redis-data
The containers created by docker-compose can be seen:
docker ps -a
They are called redis_counter_web_1 and redis_counter_myredis_1.
Cleanup
docker container rm redis_counter_myredis_1 redis_counter_web_1 docker volume rm redis_counter_redis-data
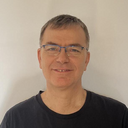
Published on 2021-04-18