How to calculate factorial in Perl - n!
I was working on some examples when I had to calculate factorial. (n! in math)
The solution without any modules:
examples/factorial.pl
use strict; use warnings; use 5.010; my $n = shift // die "Usage: $0 N\n"; my $f = 1; my $i = 1; $f *= ++$i while $i < $n; say $f;
The solution when using the reduce function of the standard List::Util module:
examples/factorial_with_reduce.pl
use strict; use warnings; use 5.010; use List::Util qw(reduce); my $n = shift // die "Usage: $0 N\n"; { my $fact = reduce { $a * $b } 1 .. $n; say $fact; } { # handles 0! = 1 as well my $fact = $n == 0 ? 1 : reduce { $a * $b } 1 .. $n; say $fact; }
reduce will take the first two values of the list on the right hand side, assign them to $a and $b respectively, execute the block.
Then it will take the result, assign it to $a and take the next element from the list, assign it to $b and execute the block. This step will be repeated till the end of the list.
This code has two versions. The first one is more simple, but does not handle 0! properly. The second works well for 0! as well.
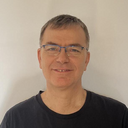
Published on 2015-04-02