false
There is no special boolean values in Perl that would mean false or true. There are certain values that evaluate to false or true in boolean context.
The values that evaluate to false in boolean context in Perl are undef, the number 0 (also when written as 0.00), the empty string, the empty array, the empty hash. In general anything that is considered to be empty. Everything else evaluates to true.
undef 0 0.00 '' '0' my @arr; my %h;
examples/boolean.pl
use strict; use warnings; use 5.010; my $x; my @arr; my %hash; if ($x) { say 'undef is true'; } else { say 'undef is false'; } if (@arr) { say 'empty array is true'; } else { say 'empty array is false'; } if (%hash) { say 'empty hash is true'; } else { say 'empty hash is false'; } my @values = (0, 0.00, '', '0', '00', "0\n", [], {}, 'true', 'false'); for my $value (@values) { if ($value) { say "$value is true"; } else { say "$value is false"; } }
The output will be:
undef is false empty array is false empty hash is false 0 is false 0 is false is false 0 is false 00 is true 0 is true ARRAY(0x55e25f1ce470) is true HASH(0x55e25f1f1080) is true true is true false is true
(When printing "0\n" the "is true" part was printed on the next row because of the newline we print.)
Boolean context
Boolean context means an if statement, and unless statement. The conditional of a while loop, a ternary operator, etc.
undef and being defined
Being "defined" or being "undef" have different meaning in Perl.
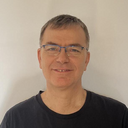
Published on 2021-03-23