How to compare version numbers in Perl and for CPAN modules
Version numbers are very flexible in Perl and unfortunately people have been abusing this freedom. even though Version numbers should be boring.
A couple of examples for version numbers:
5.010 v5.10 5.010.002 1.10 1.10_02 1.10_TEST
You might also know that 5.010 is the same as v5.10 which creates a great deal of confusion to people who are not aware of this.
There are at least two modules that handle version numbers: version and Perl::Version, but it seems only the former works properly.
So I recomment the version module.
It operator overloading to allow us to use the various numerical comparision operators such as >, < and even the spaceship operators <=> to sort a bunch of version numbers.
examples/version_example.pl
use strict; use warnings; use 5.010; use version; say version->parse( 1.23 ) < version->parse( 1.24 );
It seems to be working properly as the following cases show:
examples/version.pl
use strict; use warnings; use 5.010; use version; my @cases = ( [ 'v5.11', '5.011' ], [ 'v5.11', '5.012' ], [ '5.1.1', '5.1.2' ], [ '5.10', '5.10_01'], [ '5.10', 'v5.10'], [ '5.10', 'v5.11'], ); foreach my $c (@cases) { say '----'; say $c->[0]; say $c->[1]; say version->parse( $c->[0] ) < version->parse( $c->[1] ); say version->parse( $c->[0] ) == version->parse( $c->[1] ); }
You can also sort version numbers:
examples/sort_versions.pl
use strict; use warnings; use 5.010; use version; my @versions = ( 'v5.11', '5.011', '5.012', '5.1.1', '5.1.2', '5.10', '5.10_01'); my @sorted = sort { version->parse( $a ) <=> version->parse( $b ) } @versions; for my $s (@sorted) { say $s; }
Invalid version format
The module will not properly parse the last example:
say version->parse('1.23_TEST');
Will generate an excpetion saying Invalid version format (misplaced underscore) at ...
Comments
Hello Gabor, I'm sorting versions in my perl script and using the latest v5.10.1 but seeing the following error pop up " contains invalid data; ignoring: ", is this because of the perl version?
---
What is in the string you are parsing that gives you this error message?
---
I'm a newbie at perl in the learning process and i'm trying to sort the versions i'm passing from the @versions array and it gives the error.
--- Please show the actual source code, from this I cannot guess.
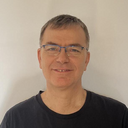
Published on 2017-08-09