Merge hashes of arrays
Given two (or more) hashes, where each value is a reference to an array how can we merge them?
Two solutions.
Merge including duplicate values
In the first one we just merge the arrays. Duplicate values remain.
examples/merge_hashes_of_arrays.pl
use 5.010; use strict; use warnings; use Data::Dump qw(dump); my %semesterOne = ( Jack => [1, 1, 2, 7], Antonie => [2, 1], ); my %semesterTwo = ( Jack => [1, 3], Antonie => [2, 4], Alex => [2, 3, 4], ); my %merged; for my $hash (\%semesterOne, \%semesterTwo) { for my $k (keys %$hash) { push @{ $merged{$k} }, @{ $hash->{$k} }; } } say dump \%merged;
examples/merge_hashes_of_arrays.txt
{ Alex => [2, 3, 4], Antonie => [2, 1, 2, 4], Jack => [1, 1, 2, 7, 1, 3] }
Merge excluding value duplication - ensuring values are unique
In the second we only keep distinct values withine each array.
examples/merge_hashes_of_arrays_uniq.pl
use 5.010; use strict; use warnings; use Data::Dump qw(dump); use List::MoreUtils qw(uniq); my %semesterOne = ( Jack => [1, 1, 2, 7], Antonie => [2, 1], ); my %semesterTwo = ( Jack => [1, 3], Antonie => [2, 4], Alex => [2, 3, 4], ); my %merged; for my $hash (\%semesterOne, \%semesterTwo) { for my $k (keys %$hash) { @{ $merged{$k} } = uniq ( @{ $merged{$k} }, @{ $hash->{$k} } ); } } say dump \%merged;
As you can see if one of the original arrays had a duplicate value (Jack in semester one had two 1-s) those will be also subject for the unification.
examples/merge_hashes_of_arrays_uniq.txt
{ Alex => [2, 3, 4], Antonie => [2, 1, 4], Jack => [1, 2, 7, 3] }
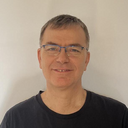
Published on 2019-05-16