our
In Perl the our keyword is used to declare one or more package variables. More exactly it creates lexical alias to a packaga variable, but for our practical purposes our means we are allowed to use the package variable without giving the fully qualified name and without violating the rules of use strict.
With that out of the way, in most cases, you'd want to declare variables in a lexical scope using the my keyword.
With our
examples/our.pl
package Person; use strict; use warnings; use 5.010; $Person::name = 'Jane'; say $Person::name; our $name; say $name;
This works printing "Jane" in both cases.
Without our
examples/without_our.pl
package Person; use strict; use warnings; use 5.010; $Person::name = 'Jane'; say $Person::name; say $name;
we get the following compilation error:
Variable "$name" is not imported at examples/without_our.pl line 9. Global symbol "$name" requires explicit package name (did you forget to declare "my $name"?) at examples/without_our.pl line 9. Execution of examples/without_our.pl aborted due to compilation errors.
Only our
examples/just_our.pl
package Person; use strict; use warnings; use 5.010; our $name = 'Bob'; say $name;
Fully qualified name
The "Fully qualified name" is when the name of a variable is preceded by the name of a package. The name of the default package in a script is called main.
Variables in the main package
In this example we have a package in its own file and it uses the fully-qualified name of a variable in the main script which is the main package to access a variable declared using our.
examples/variables_in_main.pl
use strict; use warnings; use 5.010; use CreatorOfPerl; $CreatorOfPerl::fname = 'Larry'; our $lname = 'Wall'; my $only_in_script = 'this'; CreatorOfPerl::show();examples/CreatorOfPerl.pm
package CreatorOfPerl; use strict; use warnings; use 5.010; sub show { our $fname; say $fname; # Larry say $main::lname; # Wall say $main::only_in_script; # Use of uninitialized value $only_in_script in say at examples/CreatorOfPerl.pm line 11. } 1;
perl -I examples examples/variables_in_main.pl
Other articles related to our
Check out other articles covering the our keyword. For examples scope of variables in Perl, and variable declaration in Perl.
Also check out the difference between Package variables declared with our and Lexical variables declared with my in Perl.
use strict will force you and your co-workers do declare every variable. It is a good thing. Always use strict and use warnings in your perl code!.
Check other articles about strict.
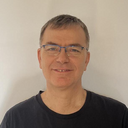
Published on 2021-02-23