$| or $OUTPUT_AUTOFLUSH - Buffering or autoflush?
If set to nonzero, forces a flush right away and after every write or print on the currently selected output channel.
By default STDOUT - the standard output is the selected channel and the default value of the $| variable is 0. That means it is buffered.
$OUTPUT_AUTOFLUSH is the name of the variable when the English module is used.
Autoflush or buffering STDOUT
In this example the $| variable is set from the command line.
examples/autoflush_stdout.pl
use strict; use warnings; my $autoflush = shift; if (defined $autoflush) { $| = $autoflush; } print "STDOUT First "; print STDERR "STDERR First "; print "STDOUT Second "; print STDERR "STDERR Second "; print "\n";
$ perl examples/autoflush_stdout.pl STDERR First STDERR Second STDOUT First STDOUT Second $ perl examples/autoflush_stdout.pl 0 STDERR First STDERR Second STDOUT First STDOUT Second $ perl examples/autoflush_stdout.pl 1 STDOUT First STDERR First STDOUT Second STDERR Second
In the first two cases, when we keep the default or explicitely set it to 0, you can see thet the 2 prints to STDERR are displayed before the 2 prints to STDOUT. That happens because STDOUT is buffered by default but STDERR is not.
In the 3rd example we set $| = 1 thereby truning the autoflush on (the buffering off). In this case every print to STDOUT immediatly shows up on the screen so the text arrives in the same order as it was sent in.
Autoflush to a file
In this example you will see how to use $| to set autoflush on a filehandle, but a better, and more modern way is to use the
use 5.010;
use strict;
use warnings;
my $filename = 'data.txt';
my $autoflush = shift;
open my $fh, '>', $filename or die;
say -s $filename; # 0
if ($autoflush) {
my $old = select $fh;
$| = 1;
select $old;
}
print $fh "Hello World\n";
say -s $filename; # 12
print $fh "Hello Back\n";
say -s $filename; # 23
close $fh;
say -s $filename; # 23
By default a filehandle is buffered. In this example we use the select keyword to change the "current default output channel",
then set $| that now works on the filehandle, finally we change the "current default output channel" back to what was earlier
which normally is the STDOUT.
First time we run it without autoflush being on and as you can see the file does not grow while we write to it only after we explicitely close the filehandle the content is written to the disk. The output channel is being buffered,
The second time we turn buffering off on the filehandle using select and $|
$ perl examples/autoflush_select.pl
0
0
0
23
$ perl examples/autoflush_select.pl 1
0
12
23
23
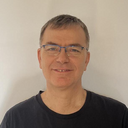
Published on 2021-04-10