Running CGI script as a Plack application with Plack::App::CGIBin
While CGI might have served the needs of your company for many years there are more advanced techniques these days. One drawback of CGI is that you usually need to have the Apache web server running it. You might also need to use mod_perl to improve the speed of your site.
This examples shows how to run them via Plackup using Plack::App::CGIBin that will make it easier to further develop your code and to run it using any modern Perl-based application server. It will also open the door and easier transition to Dancer or Mojolicious.
Our CGI "application"
We have a simple Perl script which is a CGI application. It is called app.pl and it is located in a directory called cgi-bin
We probably use it via HTTP Apache 2 server. We could even test the CGI script.
examples/cgi-bin/app.pl
use 5.010; use strict; use warnings; use CGI; my $q = CGI->new; print $q->header(-charset => 'utf-8'); my $response = ''; my $text = $q->param('text'); if (defined $text and $text =~ /\S/) { $response = qq{<hr>You sent: <b>$text</b>}; } my $html = qq{ <html> <head> <title>CGI Echo</title> </head> <body> <h1>Welcome to CGI Echo</h1> <form> <input name="text"> <input type="submit" value="Echo"> $response </form> </body> </html> }; print $html;
We can run it on the command line:
perl cgi-bin/app.pl
We can even supply it with query string parameters:
perl cgi-bin/app.pl "text=hello world"
After installing Plack::App::CGIBin we can run it as
plackup -MPlack::App::CGIBin -e 'Plack::App::CGIBin->new(root => "cgi-bin")->to_app'
In this case we can access it as http://localhost:5000/app.pl
Alternatively we can create a scrip like this:
examples/plack_app_cgibin.pl
use 5.010; use strict; use warnings; use Plack::App::CGIBin; use Plack::Builder; use FindBin; use File::Spec; my $path = File::Spec->catdir($FindBin::Bin, 'cgi-bin'); my $app = Plack::App::CGIBin->new(root => $path)->to_app; builder { mount "/cgi" => $app; };
That I place just outside the cgi-bin directory.
Here first we calculate the relative location of the cgi-bin directory (so we'll be able to run this script from any working directory).
Then we launch the application.
Finally we map it to the "/cgi" path in the URL. Just so we can see a different solution.
We can access it at this URL:
http://localhost:5000/cgi/app.pl
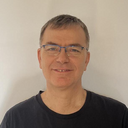
Published on 2020-12-20