List and Array Utilities in Perl
There are many utilities in perl that work on arrays or even on lists of values that have not been assigned to an array. Some of these are built in the languages. Others come in a standard module, yet even more of them can be installed from CPAN. Here you'll find a number of these utility functions and pointers where can you get them from.
Built-in functions
- grep can be used to filter values according to some rule. It usually reduces a longer list to a shorter or even empty list. In scalar context it is used to check if there is any element that fulfills certain condition.
- map can be used to transform values of a list or an array (e.g. double each number).
- sort to sort values by any condition.
- reverse returns the list of values in reverse order
- pop, push, shift, unshift to add and remove elements at either the beginning or the end of an array.
- splice to add and remove elements anywhere in an array.
- join to combine a list of values into a string.
List::Util
(checked in version 1.42 of the Scalar-List-Utils distribution.)
- reduce - Generic function to reduce a list of value to a single value according to some rule. Many other function in this module are special cases of reduce. (For example sum(@numbers) is the same as reduce { $a + $b } @numbers)
- first - like grep but will only return the first value matching the conditional in the block. The two expressions below will set the same value, but the first is faster as
it stops after it found the first element while grep has to go over all the elements before returning the results.
my $val = first { COND } @list; my ($val) = grep { COND } @list;
- max returns the element with the highest numerical value
- maxstr returns the element with the highest "string" value as returned by the gt operator.
- min returns the element with the smallest numerical value
- minstr returnes smallest "string" value.
- any is like grep in scalar context, but in addition it short-circuites making it potentially much faster. It returns true if any of the values in the given list match the supplied condition.
examples/list_util_any.pl
use strict; use warnings; use 5.010; use List::Util qw(any); my @numbers = ( 1, 2, 3, 5, 3 ); say any { $_ < 2 } @numbers; # 1 say any { $_ < 1 } @numbers; #
- all returns true if all the elements in the given list fulfill the condition. It is faster than grep as it short-circuits on the first failure.
examples/list_util_all.pl
use strict; use warnings; use 5.010; use List::Util qw(all); my @numbers = ( 1, 2, 3, 5, 3 ); say all { $_ > 0 } @numbers; # 1 say all { $_ > 1 } @numbers; #
- none - is like not all. It will return true if none of the element meet the condition. The following example shows 2 sets of 3-3 identical results:
examples/list_util_none.pl
use strict; use warnings; use 5.010; use List::Util qw(all none any); my @numbers = ( 1, 2, 3, 5, 3 ); say none { $_ < 0 } @numbers; # 1 say all { $_ >= 0 } @numbers; # 1 say not any { $_ < 0 } @numbers; # 1 say none { $_ < 2 } @numbers; # say all { $_ >= 2 } @numbers; # say not any { $_ < 2 } @numbers; #
- noall - is like not any. The following example shows 2 sets of 3-3 identical results:
examples/list_util_none.pl
use strict; use warnings; use 5.010; use List::Util qw(all none any); my @numbers = ( 1, 2, 3, 5, 3 ); say none { $_ < 0 } @numbers; # 1 say all { $_ >= 0 } @numbers; # 1 say not any { $_ < 0 } @numbers; # 1 say none { $_ < 2 } @numbers; # say all { $_ >= 2 } @numbers; # say not any { $_ < 2 } @numbers; #
- sum - sum(@numbers) - returns the sum of numbers given. For backwards compatibility, if @numbes is empty then undef is returned. Use sum0 instead!
- sum0 - Just like sum but this will return 0 if the given list was empty.
examples/list_util_sum.pl
use strict; use warnings; use 5.010; use List::Util qw(sum sum0 reduce); my @empty; my @numbers = (2, 3, 4); say sum @numbers; # 9 say sum @empty; # Use of uninitialized value in say at .. say sum0 @numbers; # 9 say sum0 @empty; # 0 say reduce { $a + $b } (0, @numbers); # 9 say reduce { $a + $b } (0, @empty); # 0
- product - multiply the numbers passed to the function. Return 1 if no value was supplied.
examples/list_util_product.pl
use strict; use warnings; use 5.010; use List::Util qw(product reduce); my @empty; my @numbers = (2, 3, 4); say product @numbers; # 24 say product @empty; # 1 say reduce { $a * $b } (1, @numbers); # 24 say reduce { $a * $b } (1, @empty); # 1
- shuffle - Returns the values of the input in a random order.
- pairs - create pairs from a list of values.
- unpairs - flatten a list of pairs into a single list of values.
- pairkeys - return a list of the odd elements of the given list
- pairvalues - return a list of the even elements of the given list
- pairgrep - it is like the built-in grep, except that it works on two elements in each iteration
- pairfirst - it is like the pairgrep, except that it returns the first hit
- pairmap - it is like the built-in map, except that it works on two elements in each iteration
List::MoreUtils
(any, all, none, notall)
- one
- apply
- insert_after
- insert_after_string
- pairwise
- mesh
- zip
- uniq
- distinct
- singleton
- after
- before
- part
- each_array
- natatime
- bsearch
- bsearchidx
- bsearch_index
- firstval
- first_value
- onlyval
- only_value,
- lastval
- last_value
- firstres
- first_result
- onlyres
- only_result
- lastres
- last_result
- indexes
- firstidx
- first_index
- onlyidx
- only_index
- lastidx
- last_index
- sort_by - a more readable, and potentially faster version of the built-in sort function.
- nsort_by - like sort_by, but compares values as numbers.
- true
- false
- minmax
List::AllUtils
Combines List::Util and List::MoreUtils in one bite-sized package.
Util::Any
Makes it easy to built list and array utilities
List::UtilsBy
- sort_by - see List::MoreUtil
- nsort_by - see List::MoreUtil
- rev_sort_by - the same as reverse sort_by ...
- rev_nsort_by - the same as reverse nsort_by ...
- min_by - the value with the smallest derivative.
- max_by
- uniq_by
- partition_by
- count_by
- zip_by
- unzip_by
- extract_by
- weighted_shuffle_by
- bundle_by
List::Pairwise
This module has a bunch of pair-wise function, but since its release List::Util was exteneded with functions providing the same service. See the list of pairwise functions of List::Util.
- mapp, map_pairwise
- grepp, grep_pairwise
- firstp, first_pairwise
- lastp, last_pairwise
- pair
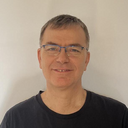
Published on 2017-06-28