Read section of a file
I was working on an example script that used a lot of calls to seek, but I kept bumping into bugs. I thought it might be a useful debugging tool to be able to read and print any section of a file given the location of the first byte and the number of bytes.
So here is a quick script I put together.
examples/read_file_section.pl
use strict; use warnings; use feature 'say'; use Fcntl qw(SEEK_SET); my ($file, $start, $length) = @ARGV; die "Usage: $0 FILE START LENGTH\n" if not $length; open my $fh, '<', $file or die; seek $fh, $start, SEEK_SET; my $buffer; read $fh, $buffer, $length; say "'$buffer'";
The seek command allows you to move the file-reading pointer back and forth in a file so you will be abel to read the file in an arbitrary order. The Fcntl module provides various constants to help you. Using the SEEK_SET constant will tell the seek command to start from the beginning of the file.
The read command allows us to read an arbitrary number of bytes from a file into a variable that people usually call a "buffer" so we used the variable name $buffer for it.
This is a sample data file:
examples/data/planets.txt
Ceres Charon Earth Jupiter Mars Mercury Neptune Pluto Saturn Uranus Venus
$ perl examples/read_file_section.pl examples/data/planets.txt 48 5 'Pluto' $ perl examples/read_file_section.pl examples/data/planets.txt 48 6 'Pluto '
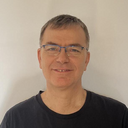
Published on 2022-09-20