Sets in Perl using Set::Scalar
In order to use sets easily in Perl we first need to install the Set::Scalar module from CPAN. You would usually use
cpanm Set::Scalar
to do this.
There are many additional ways to use the module, but let me show some of the basic examples using two sets, a few words in English and a few words in Spanish.
Method calls
We can use method calls to do operations:
examples/sets.pl
use strict; use warnings; use 5.010; use Set::Scalar; my $english = Set::Scalar->new('door', 'car', 'lunar', 'era'); my $spanish = Set::Scalar->new('era', 'lunar', 'hola'); say $english; # (car door era lunar) say $spanish; # (era hola lunar) my $in_both = $english->intersection($spanish); say $in_both; # (era lunar) my $in_both_other_way = $spanish->intersection($english); say $in_both_other_way; # (era lunar) my $in_either = $english->union($spanish); say $in_either; # (car door era hola lunar) my $english_only = $english->difference($spanish); say $english_only; # (car door) my $spanish_only = $spanish->difference($english); say $spanish_only; # (hola) my $ears_of_the_elephant = $english->symmetric_difference($spanish); say $ears_of_the_elephant; # (car door hola)
Operator overloading
We can also use overloaded operators:
examples/sets_operators.pl
use strict; use warnings; use 5.010; use Set::Scalar; my $english = Set::Scalar->new('door', 'car', 'lunar', 'era'); my $spanish = Set::Scalar->new('era', 'lunar', 'hola'); say $english; # (car door era lunar) say $spanish; # (era hola lunar) my $in_both = $english * $spanish; say $in_both; # (era lunar) my $in_both_other_way = $spanish * $english; say $in_both_other_way; # (era lunar) my $in_either = $english + $spanish; say $in_either; # (car door era hola lunar) my $english_only = $english - $spanish; say $english_only; # (car door) my $spanish_only = $spanish - $english; say $spanish_only; # (hola) my $ears_of_the_elephant = $english % $spanish; say $ears_of_the_elephant; # (car door hola)
Sets and Venn diagrams
Check out the Venn diagrams on Wikipedia if you'd like to refresh your memory or if you need to learn about sets.
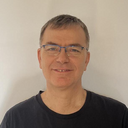
Published on 2021-02-23