Statsd with Perl
Sample scripts in Perl to use statsd. See also Statsd with Python.
examples/statsd/datadog_demo.pl
use strict; use warnings; use Net::Dogstatsd; #prints warning to STDERR if no StatsD server running on the local machine my $n = 10; for (1 .. $n) { sleep rand(2); my $elapsed_time = rand; my $dogstatsd = Net::Dogstatsd->new(); my $socket = $dogstatsd->get_socket(); $dogstatsd->increment( name => 'demo.page_views', ); $dogstatsd->gauge( name => 'demo.elapsed_time', value => $elapsed_time, ); $dogstatsd->histogram( name => 'demo.elapsed_time_histogram', value => $elapsed_time, ); }
examples/statsd/net_statsd.pl
use strict; use warnings; use Net::Statsd; # no warning even if no StatsD server running on the local machine # $Net::Statsd::HOST = 'localhost'; # Default # $Net::Statsd::PORT = 8125; # Default my $n = 10; for (1 .. $n) { sleep rand(2); my $elapsed_time = rand; Net::Statsd::increment('demo.page_views'); Net::Statsd::gauge('demo.elapsed_time' => $elapsed_time); # Net::Statsd::histogram('demo.elapsed_time_histogram' => $elapsed_time); }
examples/statsd/net_statsd_client.pl
use strict; use warnings; use Net::Statsd::Client; # no warning even if no StatsD server running on the local machine my $stats = Net::Statsd::Client->new(prefix => "demo."); my $n = 10; for (1 .. $n) { sleep rand(2); my $elapsed_time = rand; $stats->increment('page_views'); $stats->gauge('elapsed_time' => $elapsed_time); }
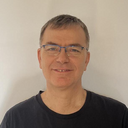
Published on 2018-10-20
If you have any comments or questions, feel free to post them on the source of this page in GitHub. Source on GitHub.
Comment on this post