use Path::Tiny instead of home-made ReadFile and WriteFile
Now that we have made the Perl code look nice, we can start cleaning up the constructs in the code and convert them to beetter expressions based in the current best practices. For this we are going to use Perl::Critic.
Running perlcritic on the tests
I ran perlcritic t/*.t which gave me a full page of output. The beginning looked like this:
t/cut.t: Bareword file handle opened at line 67, column 2. See pages 202,204 of PBP. (Severity: 5) t/cut.t: Two-argument "open" used at line 67, column 2. See page 207 of PBP. (Severity: 5) t/cut.t: Bareword file handle opened at line 77, column 2. See pages 202,204 of PBP. (Severity: 5) t/cut.t: Two-argument "open" used at line 77, column 2. See page 207 of PBP. (Severity: 5) ...
When looking at line 67 and line 77 of the t/cut.t file I saw the following:
sub ReadFile { my $file = shift; open( FILE, $file ) or return ''; local $/; undef $/; my $contents = <FILE>; close FILE; $contents; } sub WriteFile { my ( $file, $contents ) = @_; open( FILE, ">$file" ) or die "Can't open $file: $!\n"; print FILE $contents; close FILE; }
and around the file I saw rows like these:
my $content = ReadFile("filename"); ... WriteFile("filename", "content");
The ReadFile function is an implementation of the slurp-mode. It could be fixed, or it could be replaced by either File::Slurp, or by the slurp method of Path::Tiny.
Because Path::Tiny seems to be the most modern implementation I've replace the above code with the following:
use Path::Tiny qw(path);
my $content = path("filename")->slurp; ... path( "filename" )->spew( "content" );
I made similar changes to a number of other test-files.
I also had to add Path::Tiny to Makefile.PL as a prerequisite.
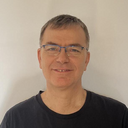
Published on 2016-02-12