Add more tests to the Markua parser
Looking at the coverage report, we can devise some additional test cases that will cover cases we thought about earlier but have not fully covered.
Theoretically in Test Driven Development (TDD) this should not happen. We are only supposed to write code that already has tests, but in reality I don't think if 100% test coverage is attainable.
I am quite sure even if it is possible, the cost/benefit ratio might not worth it.
Besides. We can easily write code that even when it has 100% test coverage it still has bugs in it.
So let's look at the details.
File Coverage / Statement Coverage
At first we look at the Statemet Coverage for our only pm file. It sais 90.9% cocerage. As we scroll down watching the 2nd column we can see 3 statements that are not covered.
The die statement on line 71. The other die statement on line 99 and the
push @errors, { row => $cnt, line => $line, }
expression on lines 146-149.
At this point none of them seems to worth the effort to write tests for. They are there to catch issues during the development. And yes, we don't need a die statement on line 146 instead of that push because our tests will fail if that line is ever executed during tests.
examples/markua-parser/bb9bc43/lib/Markua/Parser.pm
package Markua::Parser; use strict; use warnings; use Path::Tiny qw(path); our $VERSION = 0.01; # Based on https://leanpub.com/markua/read#resource-types-and-formats my $extensions = <<'EXTENSION'; txt text code Unformatted code (other) guess code Formatted code jpeg jpeg image JPEG image jpg jpeg image JPEG image png png image PNG image EXTENSION my %format; my %type; for my $line (split /\n/, $extensions) { chomp $line; my ($ext, $format, $type) = split /\s+/, $line; $format{$ext} = $format; $type{$ext} = $type; } sub new { my ($class) = @_; my $self = bless {}, $class; return $self; } sub parse_file { my ($self, $filename) = @_; my $path = path($filename); my $dir = $path->parent->stringify; my @entries; my @errors; my $cnt = 0; $self->{text} = ''; for my $line ($path->lines_utf8) { $cnt++; if ($line =~ /^(#{1,6}) (\S.*)/) { push @entries, { tag => 'h' . length($1), text => $2, }; next; } # numbered list if ($line =~ m{\A(\d+)([.\)])( {1,4}|\t)(\S.*)}) { my ($number, $sep, $space, $text) = ($1, $2, $3, $4); if (not $self->{tag}) { $self->{tag} = 'numbered-list'; $self->{list} = []; } if ($self->{tag} eq 'numbered-list') { push @{ $self->{list} }, { number => $number, sep => $sep, space => $space, text => $text, raw => $line, }; next; } die "What to do if a numbered list starts in the middle of another element?"; } # bulleted list if ($line =~ m{\A([\*-])( {1,4}|\t)(\S.*)}) { my ($bullet, $space, $text) = ($1, $2, $3); if (not $self->{tag}) { $self->{tag} = 'list'; $self->{list}{type} = 'bulleted'; $self->{list}{bullet} = $bullet; $self->{list}{space} = $space; $self->{list}{ok} = 1; $self->{list}{items} = [$text]; $self->{list}{raw} = [$line]; next; } if ($self->{tag} eq 'list') { if ($self->{list}{type} ne 'bulleted' or $self->{list}{bullet} ne $bullet or $self->{list}{space} ne $space) { $self->{list}{ok} = 0; } push @{ $self->{list}{raw} }, $line; push @{ $self->{list}{items} }, $text; next; } die "What to do if a bulleted list starts in the middle of another element?"; } # I should remember to always use \A instead of ^ even thoygh here we are really parsing lines so those two are the same if ($line =~ /\A ! \[([^\]]*)\] \(([^\)]+)\) \s* \Z/x) { my $title = $1; my $file_to_include = $2; my ($extension) = $file_to_include =~ m{\.(\w+)\Z}; if (not defined $extension or not exists $format{$extension}) { $extension = '(other)'; } my %attr = ( type => $type{$extension}, format => $format{$extension}, ); eval { my $text = path("$dir/$file_to_include")->slurp_utf8; push @entries, { tag => 'code', title => $title, text => $text, attr => \%attr, }; }; if ($@) { push @errors, { row => $cnt, line => $line, error => "Could not read included file '$file_to_include'", }; } next; } # anything else defaults to paragraph if ($line =~ /\S/) { $self->{tag} = 'p'; $self->{text} .= $line; next; } if ($line =~ /^\s*$/) { $self->save_tag(\@entries); next; } push @errors, { row => $cnt, line => $line, } } $self->save_tag(\@entries); return \@entries, \@errors; } sub save_tag { my ($self, $entries) = @_; if ($self->{tag} and $self->{tag} eq 'numbered-list') { # TODO: verify that it is a proper list for my $row (@{ $self->{list} }) { delete $row->{raw}; delete $row->{sep}; delete $row->{space}; } push @$entries, { tag => $self->{tag}, list => $self->{list}, }; $self->{tag} = undef; delete $self->{list}; return; } if ($self->{tag} and $self->{tag} eq 'list') { if ($self->{list}{ok}) { delete $self->{list}{raw}; delete $self->{list}{ok}; delete $self->{list}{space}; delete $self->{list}{bullet}; push @$entries, { tag => $self->{tag}, list => $self->{list}, }; $self->{tag} = undef; delete $self->{list}; return; } # If it is a failed list, convert it to paragraph $self->{tag} = 'p'; $self->{text} = join '', @{ $self->{list}{raw} }; delete $self->{list}; } if ($self->{tag}) { $self->{text} =~ s/\n+\Z//; push @$entries, { tag => $self->{tag}, text => $self->{text}, }; $self->{tag} = undef; $self->{text} = ''; } return; } 1; __END__ =head1 NAME Markua::Parser - Parsing Markua files and for writing books, generating DOM =head1 SYNOPSIS use Markua::Parser; my $m = Markua::Parser->new; my ($result, $errors) = $m->parse_file("path/to/file.md"); =head1 DESCRIPTION L<Markua|https://leanpub.com/markua/read> is a Markdown inspired language to write books. It was created by Peter Armstrong for L<LeanPub|https://leanpub.com/> They have an in-house partial implementation in Ruby. This is an Open Source partial implementation in Perl. The development process is described in the L<Creating a Markua Parser in Perl 5|https://leanpub.com/markua-parser-in-perl5> eBook. =head1 COPYRIGHT Copyright 2018 Gabor Szabo L<https://szabgab.com/> =head1 LICENSE This program is free software; you can redistribute it and/or modify it under the same terms as Perl 5 itself. =head1 DISCLAIMER OF WARRANTY BECAUSE THIS SOFTWARE IS LICENSED FREE OF CHARGE, THERE IS NO WARRANTY FOR THE SOFTWARE, TO THE EXTENT PERMITTED BY APPLICABLE LAW. EXCEPT WHEN OTHERWISE STATED IN WRITING THE COPYRIGHT HOLDERS AND/OR OTHER PARTIES PROVIDE THE SOFTWARE "AS IS" WITHOUT WARRANTY OF ANY KIND, EITHER EXPRESSED OR IMPLIED, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE. THE ENTIRE RISK AS TO THE QUALITY AND PERFORMANCE OF THE SOFTWARE IS WITH YOU. SHOULD THE SOFTWARE PROVE DEFECTIVE, YOU ASSUME THE COST OF ALL NECESSARY SERVICING, REPAIR, OR CORRECTION. IN NO EVENT UNLESS REQUIRED BY APPLICABLE LAW OR AGREED TO IN WRITING WILL ANY COPYRIGHT HOLDER, OR ANY OTHER PARTY WHO MAY MODIFY AND/OR REDISTRIBUTE THE SOFTWARE AS PERMITTED BY THE ABOVE LICENCE, BE LIABLE TO YOU FOR DAMAGES, INCLUDING ANY GENERAL, SPECIAL, INCIDENTAL, OR CONSEQUENTIAL DAMAGES ARISING OUT OF THE USE OR INABILITY TO USE THE SOFTWARE (INCLUDING BUT NOT LIMITED TO LOSS OF DATA OR DATA BEING RENDERED INACCURATE OR LOSSES SUSTAINED BY YOU OR THIRD PARTIES OR A FAILURE OF THE SOFTWARE TO OPERATE WITH ANY OTHER SOFTWARE), EVEN IF SUCH HOLDER OR OTHER PARTY HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. =cut # Copyright 2018 Gabor Szabo https://szabgab.com/ # LICENSE # This program is free software; you can redistribute it and/or # modify it under the same terms as Perl 5 itself.
Branch Coverage
The next level of details is looking at the Branch Coverage.
One-element numbered list
On line 60 the if ($self->{tag} eq 'numbered-list') { statement is never false. At first I thought it is because we don't have one-element numbered lists in the test cases. Accordingly I went ahead and created a test-case for a numbered list that only has a single item which, accoring to the spec, should be considered as a paragraph.
Later I found out that the test coverage report was indicating something else.
Oups.
Luckily, handling 1-element numbered lists is a special case in Markua, so we should have a test case and a proper implementation for it.
Anyway, let's see the test-case for the 1-element numbered list:
examples/markua-parser/ce64f3e/t/input/numbered-list-1-item.md
1. First line 1) First row
When I ran perl bin/generate_test_expectations.pl it generated the t/dom/numbered-list-1-item.json, but in it there were two lists. Clearly the code does not work properly. (Not surprising, I have not thought much about this example).
The solution was adding some code to the save_tag function to start to verify that the we have a proper numbered list.
Once that solution was in place, the perl bin/generate_test_expectations.pl generated the DOM I was expecting.
examples/markua-parser/ce64f3e/t/dom/numbered-list-1-item.json
[ { "tag" : "p", "text" : "1. First line" }, { "tag" : "p", "text" : "1) First row" } ]
All the other JSON files did not change so I knew I made progress.
git add . git commit -m "test case for one-element numbered list" git push
Numbered or bulleted list in another element
I've created a test case with two cases. In one switching from numbered list to bulleted list, in the second the other way around. AFAIK both should be parsed as paragraphs and both should have a error reported.
examples/markua-parser/d6064c9/t/input/switching-lists.md
1. First number * The bullet * Start with bullet 2. Then have a number
Running the DOM genrator:
perl bin/generate_test_expectations.pl
I got the following exception:
What to do if a bulleted list starts in the middle of another element? at lib/Markua/Parser.pm line 99.
Adding an error to the @errors array and converting the list to a paragraph.
I ended up with this DOM:
examples/markua-parser/d6064c9/t/dom/switching-lists.json
[ { "tag" : "p", "text" : "1. First number\n* The bullet" }, { "tag" : "p", "text" : "* Start with bullet\n2. Then have a number" } ]
and this error:
examples/markua-parser/d6064c9/t/errors/switching-lists.json
[ { "error" : "A bulleted list starts in the middle of another element.", "line" : "* The bullet\n", "row" : 3 }, { "error" : "A number list starts in the middle of another element.", "line" : "2. Then have a number\n", "row" : 7 } ]
git add . git commit -m "test and implement swiitching between list types" git push
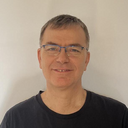
Published on 2018-04-05