Creating Makefile.PL and a CPAN distribution for the Markua Parser
When you start writing a project, especially when it is in-house, creating a CPAN distribution might not be high on your priorities, but having the capability will simplify the use of various tools and services. So I recommend that you prepare your module/application in a similar way.
We need to create a file called Makefile.PL that holds the list of dependencies of our code and a few other instructions how to install it. For simple Perl-only modules (that don't include code in C or XS or in some other language), the Makefile.PL is quite simple and standard.
examples/markua-parser/79f7a57/Makefile.PL
use strict; use warnings; use ExtUtils::MakeMaker; WriteMakefile( NAME => 'Markua::Parser', AUTHOR => q{Gabor Szabo <szabgab@cpan.org>}, VERSION_FROM => 'lib/Markua/Parser.pm', ABSTRACT => 'Parsing Markua documents and creating DOM', ( $ExtUtils::MakeMaker::VERSION >= 6.3002 ? ( 'LICENSE' => 'perl' ) : () ), PL_FILES => {}, PREREQ_PM => { 'Path::Tiny' => 0.072, 'JSON::MaybeXS' => 1, }, TEST_REQUIRES => { 'Test::More' => 1.001014, }, );
For detailed explanation see the Makefile.PL of ExtUtils::MakeMaker and the packaging with Makefile.PL articles.
The first time you run it with perl Makefile.PL you'll get a warning:
WARNING: Setting VERSION via file 'lib/Markua/Parser.pm' failed
In order to fix this we add the version number to the 'lib/Markua/Parser.pm' file:
our $VERSION = 0.01;
The whole file can be seen here:
examples/markua-parser/79f7a57/lib/Markua/Parser.pm
package Markua::Parser; use strict; use warnings; use Path::Tiny qw(path); our $VERSION = 0.01; sub new { my ($class) = @_; my $self = bless {}, $class; return $self; } sub parse_file { my ($self, $filename) = @_; my @entries; for my $line (path($filename)->lines_utf8) { if ($line =~ /^# (\S.*)/) { push @entries, { tag => 'h1', text => $1, }; } } return \@entries; } 1;
Run the tests and generate the distribution
The following sequence of command will
- Check if all the prerequisites are met and generate Makefile.
- Rearrange the files in the blib subdirectory in the same structure as they will be after installation.
- Run the tests
- Generate the MANIFEST that lists all the file that need to be included in the distribution. (This is based on the MANIFEST.SKIP file, but we did not need it for the simple case.
- Generate the tar.gz file that can be uploaded to PAUSE or distributed in another way.
$ perl Makefile.PL $ make $ make test $ make manifest $ make dist
gitignore generated files
The above process generates a few files and directories that don't need to be in version control. The best approach is to add their names to the .gitignore file so git will ignore them.
This is what I had to create:
examples/markua-parser/79f7a57/.gitignore
/MYMETA.* /Makefile /blib/ /pm_to_blib /MANIFEST /MANIFEST.bak /Markua-Parser-*
$ git add . $ git commit -m "create Makefile.PL"
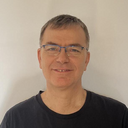
Published on 2018-03-03