ANSI command line colors with Perl
Many command on the Unix/Linux command line will print out text in various colors. For example the ls command accepts a --color flag and then it adds color to the file-system listings. You can convince your prompt to change color based on some condition. e.g. the weather outside.
How can you create such colorful output with Perl and how could you do it manually?
The key to this is the ANSI escape code tabel where you can find how to give instructions to the screen of a command-line window. Some of these instructions are related to color.
Here is an example script:
examples/colors.pl
use strict; use warnings; use feature 'say'; my $black = "\033[0;30m"; my $red = "\033[0;31m"; my $green = "\033[0;32m"; my $yellow = "\033[0;33m"; my $white = "\033[0;37m"; my $nocolor = "\033[0m"; say("Plain text in the default color"); say($green); say("Green text"); say($red); say("Red text"); say("$yellow yellow $green green $red red"); say($white); say("White text"); say($black); say("Black text");
Here I selected a few colors from the ANSI escape code table and put them in plain scalar variables. Then I only needed to print them to the screeen.
Running this script:
perl colors.pl
resulted in the following output on my computer:
Color file content manually
If I run the same script and redirect the output to a file
perl color.pl > colors.txt
I'll get a "regular" text file that looks like this if I open it with any regular editor. e.g. vim.
examples/colors.txt
Plain text in the default color [0;32m Green text [0;31m Red text [0;33m yellow [0;32m green [0;31m red [0;37m White text [0;30m Black text
However using cat to display the file will evaluate the ANSI escape codes
cat colors.txt
and you will see the same as previously when we ran the Perl script.
However you don't need a Perl script to create the file. You can edit it manually as well.
The more command gives you the same colorful result:
more colors.txt
However, these ANSI escape codes confuse the less command:
less colors.txt "colors.txt" may be a binary file. See it anyway?
less -rf and Term::ANSIColor
After posting this article I received a comment from Thomas Köhler telling me that less -r would show the color. That helped me overcome my lazyness and checked the manual page of less. I found that the -f flag would suppress the may be a binary file. See it anyway? warning. So you can use
less -rf colors.txt
He also showed two other ways to use ANSI colors in Perl.
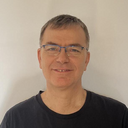
Published on 2022-09-05