Perl tutorial
About this Tutorial and eBook
The Perl Maven tutorial will teach you the basics of the Perl programming language. You'll be able to write simple scripts, analyze log files and read and write CSV files. Just to name a few common tasks.
You'll learn how to use CPAN and several specific CPAN modules.
It will be a good foundation for you to build on.
If you are interested in getting updated when new parts are published, please subscribe to the newsletter.
There is also an e-book version of the material available for purchase. In addition to the tutorial, the eBook also includes some material that is only available pro subscribers.
Supporters
Financial supporters of the Perl Tutorial
Extended eBook
An extended version of the Perl Tutorial is available as an eBook. It contains all the materials from the free tutorial and a number of additional articles that are only available to the Pro subscribers.
Brand new or Legacy?
In every tutorial and in every book there is a tension between teaching the most modern techniques or teach how the legacy code works.
The most recent techniques will only be available to people who can use the most recent version of Perl. They are probably only relevant to new projects. People who need to use older versions of Perl or who cannot install modern CPAN modules will just get frustrated seeing all the nice stuff, but not able to use it.
The old techniques on the other hand will allow you to maintain existing applications and will allow you to write new applications on both old and new versions of Perl. The drawback here is that in many cases you might need to write more code, sometimes a lot more code, than you would if you used a recent version of Perl and recent CPAN modules.
This tutorial, and this eBook tries to provide a solution to this dilemma by providing both. Telling you about the alternative options and giving advice which one to use in which situation.
Video series
There is a free video course that includes over 210 screencasts, a total of more than 5 hours of video. In addition to presenting the material it also provides explanations to the solutions of all the exercise.
There is also a free advanced Perl course.
Free on-line Beginner Perl Maven tutorial
In this tutorial you are going to learn how to use the Perl 5 programming language to get your job done.
You will learn both general language features, and extensions or libraries or as the Perl programmers call them modules. We will see both standard modules, that come with perl and 3rd-party modules, that we install from CPAN.
When it is possible I'll try to teach things in a very task oriented way. I'll draw up tasks and then we'll learn the necessary tools to solve them. Where possible I'll also direct you to some exercises you can do to practice what you have learned.
Introduction
- Install Perl, print Hello World, Safety net (use strict, use warnings)
- #!/usr/bin/perl - the hash-bang line
- Editors, IDEs, development environment for Perl
- Getting Help
- Perl on the command line
- Core Perl documentation, CPAN module documentation
- POD - Plain Old Documentation
- Debugging Perl scripts
Scalars
- Common warnings and error messages
- Prompt, read from STDIN, read from the keyboard
- Automatic string to number conversion
- Conditional statements: if
- Boolean (true and false) values in Perl
- Numerical operators
- String operators
- undef, the initial value and the defined function
- Strings in Perl: quoted, interpolated and escaped
- Here documents
- Scalar variables
- Comparing scalars
- String functions: length, lc, uc, index, substr
- Number Guessing game (rand, int)
- Perl while loop
- Scope of variables in Perl
- Boolean Short circuit
Files
- exit
- Standard Output, Standard Error and command line redirection
- warn
- die
- Writing to files
- Appending to files
- Open and read from files using Perl
- Don't open files in the old way
- Binary mode - reading and writing binary files
- eof, end of file in Perl
- tell
- seek
- truncate
- Slurp mode
Lists and Arrays
- Perl foreach loop
- The for loop in Perl
- Lists in Perl
- Using Modules
- Arrays in Perl
- Process command line parameters @ARGV
- Process command line parameters using Getopt::Long
- Advanced usage of Getopt::Long for accepting command line arguments
- split
- How to read and process a CSV file? (split, Text::CSV_XS)
- join
- The year of 19100 (time, localtime, gmtime) and introducing context
- Context sensitivity in Perl
- Reading from a file in scalar and list context
- STDIN in scalar and list context
- Sorting arrays in Perl
- Sorting mixed strings
- Unique values in an array in Perl
- Manipulating Perl arrays: shift, unshift, push, pop
- Stack
- Queue
- reverse
- The ternary operator
- Loop controls: next and last
- min, max, sum using List::Util
- qw - quote word
Subroutines
- Subroutines and Functions in Perl
- Passing multiple parameters to a function
- Variable number of parameters
- Returning a list, returning an array
- Recursive subroutines
Hashes
- Perl Hashes (dictionary, associative array, look-up table)
- Creating hash from an array
- Perl hash in scalar and list context
- exists hash element
- delete hash elements
- Sorting a hash
- Count word frequency in a text file
Regular Expressions
- Introduction to Regular Expressions in Perl
- Regex: character classes
- Regex: special character classes
- Regex: quantifiers
- Regex: Greedy and non-greedy match
- Regex: Grouping and capturing
- Regex: Anchors
- Regex options and modifiers
- Substitutions (search and replace)
- trim - remove leading and trailing spaces
- Perl 5 Regex Cheat sheet
Shell related functionality
- Perl -X operators
- Perl pipes
- Unix commands: chmod, chown, cd, mkdir, rmdir, ln, ls
- Unix commands: pwd - current working directory
- Running external programs using system
- Capturning the output of an external program using qx or backticks
- Capturing output asynchronously
- Capturing both STDOUT and STDERR
- Unix commands: rm, mv, cp: How to remove, copy or rename a file with Perl
- Windows/DOS commands: del, ren, dir
- File globbing (Wildcards)
- Directory handles
- Traversing directory tree manually with recursion
- Traversing directory tree manually using a queue and using find.
CPAN
- Download and install Perl (Strawberry Perl or manual compilation)
- Download and install Perl using Perlbrew
- Locating and evaluating CPAN modules
- Downloading and installing Perl Modules from CPAN
- How to change @INC to find Perl modules in non-standard locations?
- How to change @INC to a relative directory
- local::lib
Examples for using Perl
- How to replace a string in a file with Perl? (slurp)
- Reading Excel files using Perl
- Creating Excel files using Perl
- Sending e-mail using Perl
- CGI scripts with Perl
- Parsing XML files
- Reading and writing JSON files
- Database access using Perl (DBI, DBD::SQLite, MySQL, PostgreSQL, ODBC)
- Accessing LDAP using Perl
Common warnings and error messages
- Global symbol requires explicit package name ...
- .. also explained in Variable declaration in Perl
- Use of uninitialized value
- Bareword not allowed while "strict subs" in use
- Name "main::x" used only once: possible typo at ...
- Unknown warnings category
- Can't use string (...) as a HASH ref while "strict refs" in use at ... ...
- ... explained in Symbolic references in Perl
- Can't locate ... in @INC
- Scalar found where operator expected
- "my" variable masks earlier declaration in same scope
- Can't call method ... on unblessed reference
- Argument ... isn't numeric in numeric ...
- Can't locate object method "..." via package "1" (perhaps you forgot to load "1"?)
- Odd number of elements in hash assignment
- Possible attempt to separate words with commas
- Undefined subroutine ... called
- Useless use of hash element in void context
- Useless use of private variable in void context
- readline() on closed filehandle
- Possible precedence issue with control flow operator
- Scalar value better written as ...
- substr outside of string at ...
- Have exceeded the maximum number of attempts (1000) to open temp file/dir
- Deep recursion on subroutine
- Use of implicit split to @_ is deprecated ...
Extra articles
- Multi dimensional arrays
- Multi dimensional hashes
- Minimal requirement to build a sane CPAN package
- Statement modifiers: reversed if statements
- autovivification
- Formatted printing in Perl using printf and sprintf
From other books
Object Oriented Perl with Moose or Moo
There is a whole series of articles on writing Object Oriented code, using the light-weight Moo OOP framework or the full-blown Moose OOP framework.
There is a corresponding video courses and the whole tutorial is available as eBook.
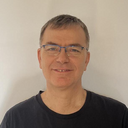
Published on 2013-07-16