Buffering or autoflush?
By default every filehandle opened for writing is buffered. We can turn of buffering (or in other words turn on autoflush) by calling the autoflush method of the filehandle.
Alternatively we can use select and set $|, $OUTPUT_AUTOFLUSH to 1 to enable autoflush.
The recommended solution is the one in this article as it makes the code more readable.
examples/autoflush.pl
use 5.010; use strict; use warnings; my $filename = 'data.txt'; my $autoflush = shift; open my $fh, '>', $filename or die; say -s $filename; # 0 if ($autoflush) { $fh->autoflush; } print $fh "Hello World\n"; say -s $filename; # 12 print $fh "Hello Back\n"; say -s $filename; # 23 close $fh; say -s $filename; # 23
By default a filehandle is buffered. In this example we use the autoflush method of the filehandle object to make it flush to the file automatically.
$ perl examples/autoflush.pl 0 0 0 23 $ perl examples/autoflush.pl 1 0 12 23 23
First time we run it without autoflush being on and as you can see the file does not grow while we write to it only after we explicitely close the filehandle the content is written to the disk. The output channel is being buffered,
The second time we turn autoflush on the filehandle and thus after every print statement we see the file growing.
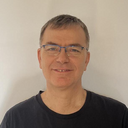
Published on 2021-04-10