Command line counter in Perl with MongoDB as storage
In the counter examples we have already seen command line solutions, but we have not used MongoDB as the back-end storage. There is a counter with MongoDB client and we are using the solution from there, but from Perl.
examples/counter_mongodb.pl
use 5.010; use strict; use warnings; use MongoDB 1.0.1; my $name = shift or die "Usage: $0 NAME\n"; my $client = MongoDB::MongoClient->new; my $db = $client->get_database( 'test' ); my $col = $db->get_collection('counter'); my $res = $col->find_one_and_update( { _id => $name }, {'$inc' => { val => 1}}, {'upsert' => 1, new => 1}, ); say $res->{val};
We assume MongoDB 1.0.1 (Version 1 introduces some changes so if you have an older version of the client this might not work.)
After connecting to the database server on localhost, accessing a database called 'test', we fetch an object to access the collection called 'counter'. As usual, we don't need to create any of these. The first time we insert any value, they will both spring to existance.
Then we call the find_one_and_update method. The first hash is the locator. It will find the document where the _id is the name we gave on the command line. The name of the counter.
The second hash is the actual update command. Here we say we would like to increment ($inc) the field 'val' by 1.
The third hash provides some additional parameters for runnin our request. upsert means we would like to update the document if it could be found, and insert it if could not be found. The new field means we would like the call to return the document after the update. (By default it would return the old version of the document.) See also the documentation of findAndModify for more options.
my $res = $col->find_one_and_update( { _id => $name }, {'$inc' => { val => 1}}, {'upsert' => 1, new => 1}, );
For further details check out the article on creating and auto-increment field.
Comments
File with same columns but -- they do not line up vertically how does one line up the columns
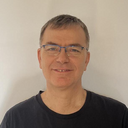
Published on 2017-05-16