Create hash from two arrays: keys and values
Given a hash we can easily create a list of all its keys and all its values by using the keys and values functions respectively.
How can we do the opposite?
How can we create a hash from an array of the future keys and an array of the future values?
Simple keys and values
examples/hash_from_two_arrays.pl
use strict; use warnings; use Data::Dumper qw(Dumper); my @keys = ('one', 'two', 'three'); my @values = ('uno', 'dos', 'tres'); my %hash; @hash{@keys} = @values; print Dumper \%hash;
What you see here is hash slices in action.
The results:
examples/hash_from_two_arrays.txt
$VAR1 = { 'three' => 'tres', 'two' => 'dos', 'one' => 'uno' };
Remember, the keys inside the hash are not in any particular order.
Values containing a list of array references
A slightly more complex case:
examples/hash_from_keys_and_array_refs.pl
use strict; use warnings; use Data::Dumper qw(Dumper); my @keys = ('name1', 'name2', 'name3'); my @values = (['name11', 'name21', 'name31'], ['name12', 'name22', 'name32'], ['name13', 'name23', undef]); my %hash; @hash{@keys} = @values; print Dumper \%hash;
And the results:
examples/hash_from_keys_and_array_refs.txt
$VAR1 = { 'name3' => [ 'name13', 'name23', undef ], 'name2' => [ 'name12', 'name22', 'name32' ], 'name1' => [ 'name11', 'name21', 'name31' ] };
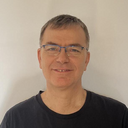
Published on 2019-05-08