.env - the dot env application configuration file
There are a number of frameworks and applications that check if there is a .env file in the root directory of the project and if there is one, then load it to enhance the content of the Environment variables.
Perl gives access to the environment variables via the %ENV hash.
As examples Docker and Laravel both make use of the file. Even IBM has it in AIX.
Apparently Philippe Bruhat (BooK) already crated a module called Dotenv that handles this.
Here is a script that uses it and then prints out the content of th whole %ENV hash and then two specific values.
examples/env/app.pl
use strict; use warnings; use Dotenv; Dotenv->load; printenv(); print("-------------------------\n"); print "$ENV{X_ANSWER}\n"; print "$ENV{X_TEXT}\n"; sub printenv { for my $key (sort keys %ENV) { printf("%-26s %s\n", $key, substr($ENV{$key}, 0, 40)); } }
Here is the config file that consists of plain key=value pairs.
examples/env/.env
X_ANSWER=42 X_TEXT = left side = right side
I use variable names starting with an X so thei will be printed last when I print the %ENV in ABC order. Otherwise there is nothing special about them.
Running
perl app.pl
will print out:
... X_ANSWER 42 X_TEXT left side = right side _ /usr/bin/perl ------------------------- 42 left side = right side
Rmember thatDoteenv will not overwrite existing keys, so if you already have an environment variable in your, well, environment, then Dotenv will keeep that value. This means you can also change the value ad-hoc when running the application:
X_ANSWER=23 perl app.pl
... X_ANSWER 23 X_TEXT left side = right side _ /usr/bin/perl ------------------------- 23 left side = right side
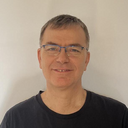
Published on 2021-04-09