my
In Perl the my keyword is used to declare one or more lexical variables. That is variables that are scoped to the enclosing block of curly braces. There are also package variables in Perl that are declared using the our keyword.
examples/my.pl
use strict; use warnings; my $this_is_global = 23; # declaring a single scalar and assigning value to it my ($x, $y, $z); # declaring multiple scalars without value, they must be enclosed in parentheses my ($person, @animals, %data); # declaring scalar, array, and hash my ($width, $length) = (23, 29); # declaring several variables and assigning value at the same time.
Check out other articles covering the my keyword. For examples scope of variables in Perl, and variable declaration in Perl.
Also check out the difference between Package variables declared with our and Lexical variables declared with my in Perl.
use strict will force you and your co-workers do declare every variable. It is a good thing. Always use strict and use warnings in your perl code!.
Check other articles about strict.
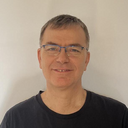
Published on 2021-02-23