Create a lexical warning that can be turned on and off with the "warnings" pragma.
Since the release of Perl 5.6 in 2000 we can and should use the warnings pragma. It allows the turning on and off of warnings in lexical blocks, that is withing any set of curly praces.
It also allows us to create our own warnings together with our own warning categories.
Warn in a module with your own category
This is how you can add a lexical warning to your code:
examples/MyMod.pm
package MyMod; use strict; use warnings; use warnings::register; sub f { my ($x) = @_; if (@_ != 1) { if (warnings::enabled()) { warnings::warn('Function f() must be called with 1 parameter! Calleed'); } } return $x+0; } 1;
Then if you call the MyMod::f function with the incorrect number of parameters, for example without any parameters, then you get a warning. (Assuming use warnings was added to your code.
You can turn off this specific warning in the whole file or in a block of code (enclosed in curly braces) with the no warnings 'MyMod'; statement as it is done in the middle of this script:
examples/mycode.pl
use 5.010; use strict; use warnings; use MyMod; { MyMod::f(); } { no warnings 'MyMod'; MyMod::f(); } { MyMod::f(); } say "done";
If we run perl mycode.pl we get the following output:
Function f() must be called with 1 parameter! Calleed at mycode.pl line 7. Use of uninitialized value $x in addition (+) at MyMod.pm line 14. Use of uninitialized value $x in addition (+) at MyMod.pm line 14. Function f() must be called with 1 parameter! Calleed at mycode.pl line 16. Use of uninitialized value $x in addition (+) at MyMod.pm line 14. done
So the 1st and 3rd calls emit our warning, but the 2nd call, where we turned off this specific warning will only emit the "uninitialized" warning.
Use existing warning categories
Instead of having your own warnnig category based on the name of the module where you registered it you can also reuse existing categories, and make your warning dependent on those.
This can be done by passing the name of that category to the warnings::enabled() function:
examples/MyMod2.pm
package MyMod2; use strict; use warnings; use warnings::register; sub f { my ($x) = @_; if (@_ != 1) { if (warnings::enabled('misc')) { warnings::warn('Function f() must be called with 1 parameter! Calleed'); } } return $x+0; } 1;
Then we turn on-off the warning using that category:
examples/mycode2.pl
use 5.010; use strict; use warnings; use MyMod2; { MyMod2::f(); } { no warnings 'misc'; MyMod2::f(); } { MyMod2::f(); } say "done";
The output then:
Function f() must be called with 1 parameter! Calleed at mycode2.pl line 7. Use of uninitialized value $x in addition (+) at MyMod2.pm line 14. Use of uninitialized value $x in addition (+) at MyMod2.pm line 14. Function f() must be called with 1 parameter! Calleed at mycode2.pl line 16. Use of uninitialized value $x in addition (+) at MyMod2.pm line 14. done
warnif
Instead of the long form:
if (warnings::enabled('cat')) { warnings::warn('text'); }
We could achieve the same using warnif:
warnings::warnif('cat', 'text');
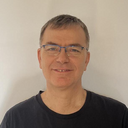
Published on 2021-06-14