Prompt for user input using IO::Prompter
In a Command Line Interface you will probably need to ask the user for input. You can do that by reading from STDIN yourself or by using a module to wrap your interaction with the user.
IO::Prompter is a module that you can use.
Simple input and input passwords
In the first example we ask the user for a simple input and then we ask for a password. The special thing in the password is that we don't want the system to echo back the characters as we type them so other people won't see them.
examples/prompter_credentials.pl
use 5.010; use strict; use warnings; use IO::Prompter; my $user = prompt 'Username:'; my $passwd = prompt 'Password:', -echo=>'*'; say $user; say $passwd;
Selector
In the second example we see how to ask the user to select an item from a list of items:
examples/prompter_selector.pl
use 5.010; use strict; use warnings; use IO::Prompter; my $selection = prompt 'Choose wisely...', -menu => [qw(wealth health wisdom)], '>'; say $selection;
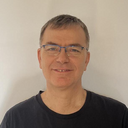
Published on 2019-04-27