Regex capturing variables $1, $2, $3, $4, $5, $6, ...
When using capturing parentheses in regualar expressins in Perl, the captures strings are available in the variables $1, $2, etc. counting the opening parenthese from left to right.
In the first example you can see two distinct pairs of parentheses. In the second example there is a third pair of parentheses around the whole regex which becomes $1 because it has the left-most opening parens.
examples/regex_capture.pl
use strict; use warnings; use 5.010; my $text = 'Some text with a number: 23 and an answer: 42 and more'; if ($text =~ /(\w+):\s+(\d+)/) { say $1; # number say $2; # 23 } if ($text =~ /((\w+):\s+(\d+))/) { say $1; # number: 23 say $2; # number say $3; # 23 }
Substitution
Capturing can also be used in the replacement part of a substitution:
examples/regex_substitute.pl
use strict; use warnings; use 5.010; my $text = '1234567890'; $text =~ s/(\d)(\d)/$2$1/g; say $text; # 2143658709
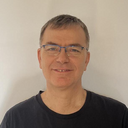
Published on 2021-03-17