Regex matching digits
For many uses only the 10 values between 0-9 are considered digits, but there are quite a few languages that have their own unicode digits.
Besides, some would consider A-F also digits. In a hexadecimal number.
So here are some regular expressions matching digits in Perl: POSIX, Unicode, ASCII - PosixDigit, Digit, PosixXDigit, [[:digit:]], [[:xdigit:]], digit, xdigit
examples/regex_for_digits.pl
use strict; use warnings; use utf8; use 5.010; binmode(STDOUT, ':utf8'); my $text = "Text with a number 42 and another number: '٣' which is 3 in arabic"; # ASCII digits say($text =~ /[0-9]/g); # 423 say($text =~ /\p{PosixDigit}/g); # 423 # Unicode digits say($text =~ /\d/g); # 42٣3 say($text =~ /[[:digit:]]/g); # 42٣3 say($text =~ /\p{Digit}/g); # 42٣3 # Hexadecimal digits say($text =~ /[[:xdigit:]]/g); # eabe42adahebec3aabc say($text =~ /[0-9a-fA-F]/g); # eabe42adahebec3aabc say($text =~ /\p{PosixXDigit}/g); # eabe42adahebec3aabc
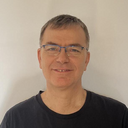
Published on 2021-03-17
If you have any comments or questions, feel free to post them on the source of this page in GitHub. Source on GitHub.
Comment on this post