Split retaining the separator or parts of it in Perl
Usually when we use split, we provide a regex. Whatever the regex matches is "thrown away" and the pieces between these matches are returned. In this example we get back the two strings even including the spaces.
examples/split_str.pl
use strict; use warnings; use Data::Dumper qw(Dumper); my $str = "abc 23 def"; my @pieces = split /\d+/, $str; print Dumper \@pieces;
$VAR1 = [ 'abc ', ' def' ];
If however, the regex contains capturing parentheses, then whatever they captured will be also included in the list of returned strings. In this case the string of digits '23' is also included.
examples/split_str_retain.pl
use strict; use warnings; use Data::Dumper qw(Dumper); my $str = "abc 23 def"; my @pieces = split /(\d+)/, $str; print Dumper \@pieces;
$VAR1 = [ 'abc ', '23', ' def' ];
Only what is captured is retained
Remember, not the separator, the substring that was matched, will be retained, but whatever is in the capturing parentheses.
examples/split_str_multiple.pl
use strict; use warnings; use Data::Dumper qw(Dumper); my $str = "abc 2=3 def "; my @pieces = split /(\d+)=(\d+)/, $str; print Dumper \@pieces;
$VAR1 = [ 'abc ', '2', '3', ' def ' ];
In this example the = sign is not in the resulting list.
Comments
That should be obvious. But it wasn’t to me. I can recall a few times I would’ve liked to retain the separators. Another tool for the tool box. Thanks!
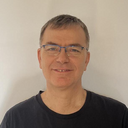
Published on 2017-10-30