Exercise: Regexes 1 - video
Regexes exercise 1
Pick up a file with some text in it. (e.g. examples/regex/text.txt ). Write a script (one for each item) that prints out every line from the file that matches the requirement. You can use the script at the end of the page as a starting point but you will have to change it!
- has a 'q'
- starts with a 'q'
- has 'th'
- has an 'q' or a 'Q'
- has a '*' in it
- starts with an 'q' or an 'Q'
- has both 'a' and 'e' in it
- has an 'a' and somewhere later an 'e'
- does not have an 'a'
- does not have an 'a' nor 'e'
- has an 'a' but not 'e'
- has at least 2 consequtive vowels (a,e,i,o,u) like in the word "bear"
- has at least 3 vowels
- has at least 6 characters
- has at exactly 6 characters
- all the words with either 'Bar' or 'Baz' in them
- all the rows with either 'apple pie' or 'banana pie' in them
- for each row print if it was apple or banana pie?
- Bonus: Print if the same word appears twice in the same line
- Bonus: has a double character (e.g. 'oo')
examples/regex_exercise_1.pl
#!/usr/bin/perl use strict; use warnings; my $filename = shift or die "$0 FILENAME\n"; open my $fh, '<', $filename or die "Could not open '$filename'\n"; while (my $line = <$fh>) { if ($line =~ /REGEX1/) { print "has an a: $line"; } if ($line =~ /REGEX2/) { print "starts with an a: $line"; } }
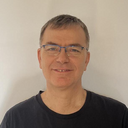
Published on 2015-07-16
If you have any comments or questions, feel free to post them on the source of this page in GitHub. Source on GitHub.
Comment on this post