Solution: sort scores - video
Solution: of the sort scores exercise.
examples/hashes/score_data.pl
#!/usr/bin/perl use strict; use warnings; my $filename = shift or die "Usage: $0 FILENAME\n"; open(my $fh, "<", $filename) or die "Could not open '$filename'\n"; my %score_of; while (my $line = <$fh>) { chomp $line; my ($name, $score) = split /,/, $line; $score_of{$name} = $score; } foreach my $name (sort keys %score_of) { printf "%-10s %s\n", $name, $score_of{$name}; } print "--------------------------\n"; foreach my $name (sort { $score_of{$b} <=> $score_of{$a} } keys %score_of) { printf "%-10s %s\n", $name, $score_of{$name}; }
We run it as perl score_data.pl score_data.txt.
examples/hashes/score_data.txt
Foo,23 Bar,70 Baz,92 Bozo,17 Gozo,52 Dardon,20 Mekodra,23
The code starts with the usual hash-bang line followed by use strict; and use warnings;.
Then we get the name of the data file from the command line using shift.
Then we open the file for reading.o
Then we declare a hash called %score_of in which we are going to hold the name-value pairs from the data file.
We go over the file line-by-line using a while loop. chomp off the newline from the end of the line and the split at the comma (,).
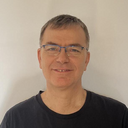
Published on 2015-07-09