Dancer 1 echo using POST and testing POST
In this example we'll see how to accept POST parameters in a Dancer 1 application. We'll also see how to write tests for it.
Directory Layout
. ├── bin │ └── app.pl ├── lib │ └── App.pm └── t └── 01-index.t
Code launching the app
examples/dancer/app4/bin/app.pl
#!/usr/bin/env perl use Dancer; use App; dance;
The code of the application
examples/dancer/app4/lib/App.pm
package App; use strict; use warnings; use Dancer ':syntax'; get '/' => sub { return q{ <h1>Echo with POST</h1> <form action="/echo" method="POST"> <input type="text" name="txt"> <input type="submit" value="Send"> </form> }; }; post '/echo' => sub { return 'You sent: ' . param('txt'); }; true;
The test code
examples/dancer/app4/t/01-index.t
use Test::More tests => 2; use strict; use warnings; # the order is important use App; use Dancer::Test; subtest index => sub { my $resp = dancer_response GET => '/'; is $resp->status, 200; like $resp->content, qr{<h1>Echo with POST</h1>}; like $resp->content, qr{<form}; }; subtest echo_post => sub { my $resp = dancer_response POST => '/echo', { params => { txt => 'Hello World!', } }; is $resp->status, 200; is $resp->content, 'You sent: Hello World!'; };
The call to dancer_response returns a Dancer::Respons object. Feel free to use its methods.
Running the tests
Be in the root directory of your project. (The common parent directory of bin, lib, and t.) and type:
prove -l
or
prove -lv
for more verbose output.
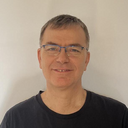
Published on 2019-04-25
If you have any comments or questions, feel free to post them on the source of this page in GitHub. Source on GitHub.
Comment on this post