Dancer 1 test Hello World
Writing web applications can be fun. Adding more features can be more fun.
Making mistakes is not fun.
Introducing bugs in parts of the application that was already running is not fun.
Wrting test is fun. Or at least helps you avoid bugs.
Directory Layout
. ├── bin │ └── app.pl ├── lib │ └── App.pm └── t └── 01-index.t
Code launching the app
examples/dancer/app2/bin/app.pl
#!/usr/bin/env perl use Dancer; use App; dance;
The code of the application
examples/dancer/app2/lib/App.pm
package App; use strict; use warnings; use Dancer ':syntax'; get '/' => sub { return 'Hello World'; }; true;
The test code
examples/dancer/app2/t/01-index.t
use Test::More tests => 2; use strict; use warnings; # the order is important use App; use Dancer::Test; my $resp = dancer_response GET => '/'; is $resp->status, 200; is $resp->content, 'Hello World';
The call to dancer_response returns a Dancer::Respons object. Feel free to use its methods.
Running the tests
Be in the root directory of your project. (The common parent directory of bin, lib, and t.) and type:
prove -l
or
prove -lv
for more verbose output.
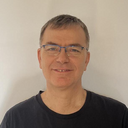
Published on 2019-04-25