How to create an Indian Rupee symbol with Perl code
Recently I got an e-mail from one of the readers asking how to create a Indian Rupee symbol with Perl. I told him to check what is the Unicode character for the Rupee symbol and then to check how to print Unicode characters with Perl.
As this might be interesting to more people, let me show it here.
The first search led me to the Wikipedia page describing the Indian Rupee sign.
Apparently there is a Generic Rupee sign U+20A8 ₨ and a specific Indian Rupee sign U+20B9 ₹.
If you'd like to print a Unicode character to the screen (standard output or STDOUT), then you need to tell Perl to change the encoding of the STDOUT channel to UTF8. You can do this with the binmode function. (Check out the Unicode intro for further details.)
For the specific code points you'd use the \x sign and then put the Hexadecimal values, in our case 20A8 and 20B9 respectively in curly braces.
use strict; use warnings; use 5.010; binmode(STDOUT, ":utf8"); say "\x{20A8}"; # ₨ say "\x{20B9}"; # ₹
Of course if you are creating an HTML page then you'd better include the HTML entity representing the same character which is an at sign (&) followed by a pound sign (#), followed by the decimal representation of the code point, followed by a semi-colon. To convert the Hexadecimal values to decimal values you can do the following in perl:
print 0x20A8, "\n"; # 8360 print 0x20B9, "\n"; # 8377
So in HTML you would include ₨ and ₹ respectively.
I have used perl 5.14.2 on Linux to test the above. In older versions of Perl the above might not work.
examples/rupee.pl
use strict; use warnings; use 5.010; # http://en.wikipedia.org/wiki/Indian_rupee_sign # # U+20A8 # U+20B9 binmode(STDOUT, ":utf8"); say "\x{20A8}"; say "\x{20B9}"; say 0x20A8; say 0x20B9; my $generic = "20A8"; say $generic; say hex $generic; # 8360
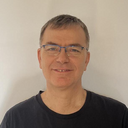
Published on 2013-03-16