Returning multiple values or a list from a subroutine in Perl
It is really easy to return multiple values from a subroutine in Perl. One just needs to pass the values to the return statement.
Returning multiple values from a function in Perl
Perl will convert the values into a list that the caller capture in a number of ways. In the first call the values are captured in an array. In the second we capture them in a list of scalar variables. Of course you can even mix these two approaches if that fits your application.
examples/return_multiple_elements.pl
use strict; use warnings; use Data::Dumper qw(Dumper); sub compute { my ($x, $y) = @_; my $add = $x + $y; my $multiply = $x * $y; my $subtract = $x - $y; my $divide = $x / $y; return $add, $multiply, $subtract, $divide; } my @result = compute(42, 2); print Dumper \@result; my ($sum, $mul, $sub, $div) = compute(102, 3); print "sum: $sum\n"; print "mul: $mul\n"; print "sub: $sub\n"; print "div: $div\n";
The result will look like this:
$VAR1 = [ 44, 84, 40, '21' ]; sum: 105 mul: 306 sub: 99 div: 34
Returning a an array from a function in Perl
In this example we compute the first N element of the Fibonacci series. Collect them in an array inside the function and then return the array.
Behind the scenes Perl sees the content of this array and returns the elements as a list.
Usually the caller will assign the result to an array as we did in the first call of fibonacci(8). This is usually the logical thing to do.
However there is nothing in Perl that would stop us from capturing some of the returned values in individual scalar as it is done in the second example when calling fibonacci(6).
examples/fibonacci_list.pl
use 5.010; use strict; use warnings; use Data::Dumper; sub fibonacci { my ($n) = @_; if ($n == 1) { return 1; } if ($n == 2) { return 1, 1; } my @fib = (1, 1); for (2 .. $n) { push @fib, $fib[-1] + $fib[-2]; } return @fib; } my @results = fibonacci(8); print Dumper \@results; my ($first, $second, @rest) = fibonacci(6); print "first: $first\n"; print "second: $second\n"; print Dumper \@rest;
$VAR1 = [ 1, 1, 2, 3, 5, 8, 13, 21, 34 ];first: 1 second: 1 $VAR1 = [ 2, 3, 5, 8, 13 ];
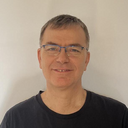
Published on 2021-08-15