trim - removing leading and trailing white spaces with Perl
In some other languages there are functions called ltrim and rtrim to remove spaces and tabs from the beginning and from the end of a string respectively. Sometimes they even have a function called trim to remove white-spaces from both ends of a string.
There are no such functions in Perl (though I am sure there are plenty of CPAN modules implementing these functions) as a simple substitution regex can solve this.
Actually it is so simple that it is one of the great subjects of bike-shedding
left trim
ltrim or lstrip removes white spaces from the left side of a string:
$str =~ s/^\s+//;
From the beginning of the string ^ take 1 or more white spaces (\s+), and replace them with an empty string.
right trim
rtrim or rstrip removes white spaces from the right side of a string:
$str =~ s/\s+$//;
Take 1 or more white spaces (\s+) till the end of the string ($), and replace them with an empty string.
trim both ends
trim remove white space from both ends of a string:
$str =~ s/^\s+|\s+$//g;
The above two regexes were united with an an alternation mark | and we added a /g at the end to execute the substitution globally (repeated times).
Hiding in function
If you really don't want to see those constructs in your code you can add these functions to your code:
sub ltrim { my $s = shift; $s =~ s/^\s+//; return $s }; sub rtrim { my $s = shift; $s =~ s/\s+$//; return $s }; sub trim { my $s = shift; $s =~ s/^\s+|\s+$//g; return $s };
and use them like this:
my $z = " abc "; printf "<%s>\n", trim($z); # <abc> printf "<%s>\n", ltrim($z); # <abc > printf "<%s>\n", rtrim($z); # < abc>
String::Util
If you really, really don't want to copy that, you can alway install a module.
For example String::Util provides a function called trim that you can use like this:
use String::Util qw(trim); my $z = " abc "; printf "<%s>\n", trim( $z ); # <abc> printf "<%s>\n", trim( $z, right => 0 ); # <abc > printf "<%s>\n", trim( $z, left => 0 ); # < abc>
By default it trims on both sides and you have to turn off trimming. I think, having your own ltrim and rtrim will be clearer.
Text::Trim
Another module, one that provides all the 3 function is Text::Trim, but it take the Perl-ish writing a step further, and maybe to slightly dangerous places.
If you call it and use the return value in a print statement or assign it to a variable, it will return the trimmed version of the string and will keep the original intact.
use Text::Trim qw(trim); my $z = " abc "; printf "<%s>\n", trim($z); # <abc> printf "<%s>\n", $z; # < abc >
On the other hand, if you call it in VOID context, that is when you don't use the return value at all, the trim function will change its parameter, in a way similar to the behavior of chomp.
use Text::Trim qw(trim); my $z = " abc "; trim $z; printf "<%s>\n", $z; # <abc>
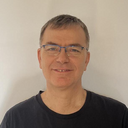
Published on 2013-04-12