Moo with hash reference as attributes - with or without default values
TL;DR use: default => sub { {} }
Moo can also have attributes where the value is a HASH reference and you might want to ensure that even if the user has not supplied an HASH reference at the construction time, the attribute still has an empty hash. So you write this code:
has children => (is => 'rw', default => {});
When you try to create a new Person object in the programming.pl script:
examples/moo_with_hash_bad.pl
package Person; use Moo; has children => (is => 'rw', default => {}); package main; use strict; use warnings; use 5.010; #use Person; my $joe = Person->new; say $joe->children; my $pete = Person->new; say $pete->children;
We get the following exception:
Invalid default 'HASH(0x56377da51780)' for Person->children is not a coderef or code-convertible object or a non-ref at ...
The fix is to wrap the default creation in an anonymous subroutine:
has children => (is => 'rw', default => sub { {} });
examples/moo_with_hash_good.pl
package Person; use Moo; has children => (is => 'rw', default => sub { {} }); package main; use strict; use warnings; use 5.010; #use Person; my $joe = Person->new; say $joe->children; my $pete = Person->new; say $pete->children;
And the output looks like this:
HASH(0x55bfd7e64e88) HASH(0x55bfd7e65230)
Two different HASH references.
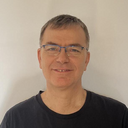
Published on 2020-07-31