Beginner Perl Maven video course - video
You can watch the episodes on YouTube, or on this site. Some of the pages here have extra text.
Table of Contents
1. Introduction
- Welcome and installation
- Editors, IDEs
- Hello World
- Safety net (use strict; use warnings;)
- Debugging
- Comments
- Documentation of Perl
- POD - Plain Old Documentation
- Perl on the command line
- Exercise: Hello World
- Solution: Hello World (part 1)
- Solution: Hello World (part 2)
2. Scalars
- Scalar values and variables
- Common Errors
- Variable interpolation - User Input and Output - chomp
- Numerical Operators
- String Operators
- If statement
- Comparison Operators
- String to Number Conversion
- Compare Values
- undef
- Logical Operators
- True and False values
- Short circuit
- String functions: index
- Substr
- String in Double quotes
- String in Single quotes
- Scope of Variables
- rand() and int()
- Here documents
- Exercise: rectangular
- Solution: Area of rectangular
- Solution: Area prompt and warn if values are less than 0
- Solution: Concatenation
- Exercise: Number guessing game
- Solution: Number guessing game
3. Files
- die, warn, exit
- Open File
- Open File All
- Open File with Error Handling
- open or die
- No such file
- Read one line
- While loop
- Read file line by line
- Write to file
- Sum numbers in a file
- Debug the sum numbers script
- Analyze Apache log file
- Old Style Open
- Exercise: Add more statistics
- Solution: Add more statistics
- Exercise: Write report to file
- Solution: Write report to file
4. Lists and Arrays
- Lists in Perl
- List Assignment
- Foreach Loop Over List
- Creating and Array
- Array Assignment
- Exercise: more statistics
- Solution: more statistics (placeholder)
- Array indexes
- Array indexes (screencast)
- Looks like number
- Command line parameters
- Command line parameters (screencast)
- Processing command line using Getopt::Long
- Processing command line using Getopt::Long (screencast)
- Process CSV file
- Process CSV file - short version
- One-liner - Sum of values in CSV file
- Process CSV file - using Text::CSV_XS
- Join
- Exercise: improve the color selector
- Solution: improve the color selector
- Solution: improve the color selector - check input
- Solution: improve the color selector - force
- Solution: improve the color selector - colors.txt
- Solution: improve the color selector - --filename
- Exercise: improve the number guessing game
- Solution: improve the number guessing game - multiple guesses
- Solution: improve the number guessing game - exit
- Solution: improve the number guessing game - s for show
- Solution: improve the number guessing game - n for next game
- Solution: improve the number guessing game - d for debug mode
- Solution: improve the number guessing game - m for move mode
5. Advanced Arrays
- The Year of 19100
- Array in Context
- Context Sensitivity
- Filehandle in SCALAR and LIST context
- Slurp
- Pop and Push
- Loop controls: next and last
- shift and unshift
- shift
- reverse
- sort
- Ternary operator
- Count Digits
- Exercise: Display unique rows of a file
- Exercise: sort mixed string
6. Functions and Subroutines
7. Hashes
- A hash and its uses
- Creating a hash
- Creating a hash from an array
- Hash in scalar context
- Fetching data from hash
- Exists and Delete in hash
- Counting words in a file
- Dumping hash
- Exercise: parse HTTP values
- Solution: parse HTTP values
- Exercise: Improve color selector
- Solution: Improve color selector
- Exercise: Sort scores
- Solution: Sort scores
- Exercise: Improve Apache log analyzer
- Solution: Improve Apache log analyzer
- Exercise: Parse variable width fields
- Solution: Parse variable width fields
8. Regular Expressions - part 1
- Regexes
- Where can I use regexes
- Simple use of regex
- Finding a string in a file
- Single character match
- Match any character
- Character classes
- Negated character class
- Optional characters
- Any Number of any Characters
- Quantifiers
- Quantifiers on Character classes
- Exercise: Regexes 1
- Solution: Regexes 1
- Solution: Regexes 2
- Solution: Regexes 3
9. Regular Expressions - part 2
- Regex Alternatives
- Regex Capturing
- Regex Anchors
- More about Character classes
- Special character classes
- Exercise: Match numbers with regex
- Solution: Match numbers with regex
- Exercise: hexa, octal, binary
- Solution: hexa, octal, binary
- Exercise: Roman numbers
10. Regular Expressions - part 3
- m/ for matching regexes
- Case insensitive regexes using /i
- multiple lines in regexes using /m
- Single line regexes using /s
- /x modifier for verbose regexes
- Substitution
- Global substitution with regexes
- Greedy regex quantifiers
- Minimal Regex Matching
- trim
- Fixing assembly with Perl
- split with regex
- Fixing dates using Regexes
- Exercise split HTTP using Regexes
- Solution: split HTTP using Regexes
- Exercise: split path - filename/dirname
- Solution: split path - filename/dirname
- Exercise: sort SNMP numbers
- Solution: sort SNMP numbers
- Exercise: parse hours log file and create time report
- Solution: parse hours log file and create time report (placeholder)
- Exercise: parse INI file
- Solution: parse INI file (placeholder)
- Exercise: parse Perl file
- Solution parse Perl file (placeholder)
11. Shell to Perl
- shell -x
- Running external programs
- Unix and DOS commands
- file globbing
- rename files
- directory handle
- File::HomeDir
- more Unix commands
- File::Spec
- File::Find
12. CPAN
- Using procedural module
- Using object oriented module
- Changing @INC
- Changing @INC relative path
- What is CPAN
- Some interesting CPAN modules
- Installing modules from the OS vendor
- Installing Perl modules with cpan
- search.cpan.org
- CPAN Testers and CPAN Ratings
13. Applications
- Create Linux user account
- Diskspace usage: df in Perl
- Reporting diskspace usage on mail server
- disk usage: du
- Send email with attachments
- Reading Excel file
- read fixed-width records
- Processing config file
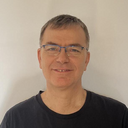
Published on 2012-07-06
If you have any comments or questions, feel free to post them on the source of this page in GitHub. Source on GitHub.
Comment on this post