Dancer 1 echo using GET and testing GET
In this example we'll see how to accept GET parameters in a Dancer 1 application. We'll also see how to write tests for it.
Directory Layout
. ├── bin │ └── app.pl ├── lib │ └── App.pm └── t └── 01-index.t
Code launching the app
examples/dancer/app3/bin/app.pl
#!/usr/bin/env perl use Dancer; use App; dance;
The code of the application
examples/dancer/app3/lib/App.pm
package App; use strict; use warnings; use Dancer ':syntax'; get '/' => sub { return q{ <h1>Echo with GET</h1> <form action="/echo" method="GET"> <input type="text" name="txt"> <input type="submit" value="Send"> </form> }; }; get '/echo' => sub { return 'You sent: ' . param('txt'); }; true;
The test code
examples/dancer/app3/t/01-index.t
use Test::More tests => 3; use strict; use warnings; # the order is important use App; use Dancer::Test; subtest index => sub { my $resp = dancer_response GET => '/'; is $resp->status, 200; like $resp->content, qr{<h1>Echo with GET</h1>}; like $resp->content, qr{<form}; }; subtest echo_get_1 => sub { my $resp = dancer_response GET => '/echo?txt=Hello World!'; is $resp->status, 200; is $resp->content, 'You sent: Hello World!' }; subtest echo_get_2 => sub { my $resp = dancer_response GET => '/echo', { params => { txt => 'Hello World!', } }; is $resp->status, 200; is $resp->content, 'You sent: Hello World!'; };
The call to dancer_response returns a Dancer::Respons object. Feel free to use its methods.
After testing the index page there are two examples for passing parameters in the test. The first one uses the same syntaxt you'd see on a URL, in the second one we let Dancer constuct the URL. Use whichever fits your workflow.
Running the tests
Be in the root directory of your project. (The common parent directory of bin, lib, and t.) and type:
prove -l
or
prove -lv
for more verbose output.
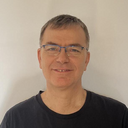
Published on 2019-04-25