Appending to files
In this episode of the Perl tutorial we are going to see how to append to files using Perl.
In the previous episode we learned how to write to files. That's good when we are creating a file from scratch, but there are cases when you would rather keep the original file, and only add lines to the end.
The most prominent case is when you are writing a log file.
This article shows how to do this using core perl function. There is a more modern and more readable way using Path::Tiny.
Calling
open(my $fh, '>', 'report.txt') or die ...
Opening a file for writing using the > sign will delete the content of the file if it had any.
If we would like to append to the end of the file we use two greater-than signs >> as in this example:
open(my $fh, '>>', 'report.txt') or die ...
Calling this function will open the file for appending. that means the file will remain intact and anything your print() or say() to it will be added to the end.
The full example is this:
use strict; use warnings; use 5.010; my $filename = 'report.txt'; open(my $fh, '>>', $filename) or die "Could not open file '$filename' $!"; say $fh "My first report generated by perl"; close $fh; say 'done';
If you run this script several times, you will see that the file is growing. Every run of the script will add another row to the file.
Prepending to the beginning of a file
In case you'd like to the top of the file there is another article explaning how to write to the beginning of a file.
Comments
Now, How do we append/write to the top of the file? not the end
--- You cannot. You need to rewrite the whole file.
--- Here is a new article explaining it https://perlmaven.com/how-to-write-to-the-beginning-of-a-file
--- Thanks, I got it figured out. I found my old code from 10 years ago where I made a blogger back before I started using wordpress. Thanks for the article. I give it a read, I forgot how to do anything in perl, but it's coming back to me.
Hello, I need to append 2 Hex files and create a new hex file. Used the following code, it is printing to a new file, however only 1495 lines out of 1545 lines are copied to third file from second file and not copy pasting any content from the first file. Both the input files are of different size. Kindly help me to resolve this issue.
The Perl script used:
my @files = ("02316-0493-0001.hex", "02316-0494-0002.hex"); my @fhs;
foreach my $file (@files) { open($fh, $file) || die; push(@fhs, $fh); } open(MIXED, ">mixed.hex") || die; while (1) { foreach $fh (@fhs) { $line = <$fh>; last if (eof($fh)); print MIXED $line; } } map { close($_); } @fhs; close(MIXED);
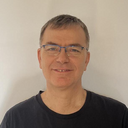
Published on 2013-03-15