Conditional statements, using if, else, elsif in Perl
So far in the tutorial each statement got executed one after the other. There are cases when we want to execute certain statements only if some condition is met: i.e. if the condition evaluates to "true".
The if statement is used for this task.
The basic structure of the if-statement is
if (CONDITION) { STATEMENT; ... STATEMENT; }
For example
examples/input_and_condition.pl
use strict; use warnings; print "What is your age? "; my $age = <STDIN>; if ($age >= 18) { print "In most countries you can vote.\n"; }
Both the parentheses around the condition and the curly braces {} are required. (A special note for people with background in the C programming language: Even if there is only one statement, that statement needs to be wrapped in a pair of curly braces.)
The condition itself can be any Perl-expression. If it is true, the statement(s) inside the curly braces will be executed. If it is false, it will be skipped. (For what constitutes true and false, check the article about boolean values.)
Layout: Indentation
As in Perl white-spaces are not significant we could have laid-out the code in a number of different ways, without any impact on what the code does, but let me point out the way you see it here and why it is the recommended layout. The important part is that the code inside the curly braces does not start at the beginning of the line. It starts a few characters to the right. This is called indentation. In the tutorial we will see a number of statements that have an internal block (a pair of curly braces), and in each one of those we'll indent the code to the right. This indentation makes it easier for the reader to see which parts of the code is inside the block and what is outside. There are languages, such as Python that force you to add such indentation. Perl is much more laid-back, and much more flexible in this area, but it is still strongly recommended that you always indent your code.
People love to debate whether one should use a tab character or spaces for indentation, and the "spaces" camp is also subdivided by the number of spaces. I think it is not the critical part. The important thing is that the code will be indented in a consistent way.
In these examples I use 4 spaces, but when I write an application I usually use TAB characters. When I have to edit the code written by other people, I try to follow their style. If there is one.
if - else
There are cases when we want to do something if a condition is met (when it is true), and something else if it is false. For this we can use the else extension of the if-statement:
examples/if_else.pl
use strict; use warnings; print "What is your age? "; my $age = <STDIN>; if ($age >= 18) { print "In most countries you can vote.\n"; } else { print "You are too young to vote\n"; }
In this code, if the condition was true and $age was greater or equal to 18, then the if-block was executed. If the condition was false then the else-block was executed.
As we can see from the first example, the else-part is optional, but if we add the word else we also have to add the curly braces.
Nested if statements
examples/nested_statements.pl
use strict; use warnings; print "What is your age? "; my $age = <STDIN>; if ($age >= 18) { print "In most countries you can vote.\n"; if ($age >= 23) { print "You can drink alcohol in the USA\n"; } } else { print "You are too young to vote\n"; if ($age > 6) { print "You must go to school...\n"; } }
Perl allows to add addition if-statements, both in the if-block and in the else-block.
Note, the code inside the internal blocks is further indented to the right with another 4 spaces.
elsif
In another example we can see how we can easily have several levels of indentation:
examples/deep_indentation.pl
use strict; use warnings; print "What is your age? "; my $age = <STDIN>; if ($age < 6) { print "You are before school\n"; } else { if ($age < 18) { print "You must go to school\n"; } else { if ($age < 23) { print "In most countries you can vote.\n"; } else { print "You can drink alcohol in the USA\n"; } } }
This works properly, but it has two issues:
One is that the width of the screen is limited. If you indent too much then either your rows will be too wide and won't fit in the screen or you'll have to wrap the code. Both make it harder to read the code.
The second one is that most programmers when reading code tend to focus on what's on the left hand side and either neglect the right hand side of the code or just perceive as less important. So by indenting the code you send the message to the maintenance programmer, that the nested conditions are less important. If this is your intention then go ahead write the code this way, on the other hand, if the only reason to write nested conditions is because that's the easy order to evaluate the conditions, then you'd be better off using elsif and flattening the construct.
examples/flat_indentation.pl
use strict; use warnings; print "What is your age? "; my $age = <STDIN>; if ($age < 6) { print "You are before school\n"; } elsif ($age < 18) { print "You must go to school\n"; } elsif ($age < 23) { print "In most countries you can vote.\n"; } else { print "You can drink alcohol in the USA\n"; }
This code does exactly as the previous one, but this time, instead of nesting an if-statement inside the else-block, we used an elsif-statement which means we don't need an extra indentation level. This also sends the message that the 4 cases have the same importance.
When you code, remember, the computer will understand you either way, but make it easy for the next reader to understand your intentions. Who knows, that person might be you...
else if in Perl
Please also note that the spelling of the "else if" construct has the most diversity among programming languages. In Perl it is written in one word with a single 'e': elsif.
Empty block
Though usually it is not necessary, in Perl we can also have empty blocks:
examples/empty_block.pl
use strict; use warnings; print "What is your age? "; my $age = <STDIN>; if ($age < 0) { }
Sometimes we write this when first we write the skeleton of the program and only later add the code that needs to be in the block.
We can add comments inside, but we don't have to.
examples/empty_block_comment.pl
use strict; use warnings; print "What is your age? "; my $age = <STDIN>; if ($age < 0) { # TODO }
Actually, there is even a special code-snippet that was added to version 5.10 of perl:
examples/block_with_yada_yada.pl
use strict; use warnings; print "What is your age? "; my $age = <STDIN>; if ($age < 0) { ... }
The 3 dots there, is called the yada, yada operator. It is what most people would write when they are jotting down some text and want to leave place for more. Some might write etc.
Since version 5.10 came out, it is also a valid construct in Perl. It will throw an Unimplemented exception if it is executed. It can be very handy, but probably a bit premature to learn so early in the Perl Tutorial.
Comments
Thanks for the nice tutorials! I'm attempting to write a small perl script and it seems my searches keep leading me to different pages here. It must have taken quite awhile to put all this together...
--- Thanks. It took a few years.
Yes, thank you very much for this guide. It's quite helpful.
Enjoying your website. Still more to come. Thanks for the great PERL tutorial.
can you give me the example of if - elsif with -- this statement -- do you want to continue if yes then program ask same question like what is age. if we select no then program exist. thanks in advance
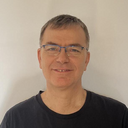
Published on 2014-04-22