delete an element from a hash
The delete function will remove the given key from a hash.
examples/delete.pl
#!/usr/bin/perl use strict; use warnings; use Data::Dumper qw(Dumper); my %phones = ( Foo => '111', Bar => '222', Moo => undef, ); print exists $phones{Foo} ? "Foo exists\n" : "Foo does not exist\n"; print Dumper \%phones; delete $phones{Foo}; print exists $phones{Foo} ? "Foo exists\n" : "Foo does not exist\n"; print Dumper \%phones;
At first the key "Foo" exists in the hash and after calling delete it does not exist any more.
Result:
Foo exists $VAR1 = { 'Foo' => '111', 'Moo' => undef, 'Bar' => '222' }; Foo does not exist $VAR1 = { 'Moo' => undef, 'Bar' => '222' };
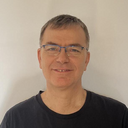
Published on 2021-09-30
If you have any comments or questions, feel free to post them on the source of this page in GitHub. Source on GitHub.
Comment on this post