min, max, sum in Perl using List::Util
The List::Util module provides a number of simple and some more complex functions that can be used on lists, anything that returns a list anything that can be seen as a list.
For example these can be used on arrays as they "return their content" in list context.
min
If given a list of numbers to it, it will return the smallest number:
examples/min.pl
use 5.010; use strict; use warnings; use List::Util qw(min); say min( 10, 3, -8, 21 ); # -8 my @prices = (17.2, 23.6, 5.50, 74, '10.3'); say min(@prices); # 5.5 # Argument "2x" isn't numeric in subroutine entry at examples/min.pl line 14. say min( 10, 3, '2x', 21 ); # 2
If one of the arguments is a string that cannot be fully converted to a number automatically and if you have use warnings on as you should, then you'll see the following warnings: Argument ... isn't numeric in subroutine entry at ...
minstr
There is a corresponding function called minstr that will accept strings and sort them according to the ASCII order, though I guess it will work with Unicode as well if that's what you are feeding it.
examples/minstr.pl
use 5.010; use strict; use warnings; use List::Util qw(minstr); say minstr( 'f', 'b', 'e' ); # b
It can also accept numbers as parameters and will treat them as strings. The result might surprise you, if you are not familiar with the automatic number to string conversion of Perl, and that the string "11" is ahead of the string "2" because the comparison works character-by-character and in this case the first character of "11" is ahead of the first (and only) character of "2" in the ASCII table.
examples/minstr_numbers.pl
use 5.010; use strict; use warnings; use List::Util qw(minstr); say minstr( 2, 11, 99 ); # 11
After all internally it uses the lt operator.
max
Similar to min just returns the biggest number.
maxstr
Similar to minstr, returns the biggest string in ASCII order.
sum
The sum function adds up the provided numbers and returns their sum. If one or more of the values provided is a string that cannot be fully converted to a number it will generate a warning like this: Argument ... isn't numeric in subroutine entry at .... If the parameters of sum are empty the function returns undef. This is unfortunate as it should be 0, but in order to provide backwards compatibility, if the provided list is empty then undef is returned.
examples/sum.pl
use 5.010; use strict; use warnings; use List::Util qw(sum); say sum( 10, 3, -8, 21 ); # 26 my @prices = (17.2, 23.6, '1.1'); say sum(@prices); # 41.9 my @empty; # Use of uninitialized value in say at examples/sum.pl line 14. say sum(@empty); # (prints nothing)
sum0
In order to fix the above issue, that sum() return undef, in version 1.26 of the module, in 2012, a new function called sum0 was introduced that behaves exactly like the sum function, but returns 0 if no values was supplied.
examples/sum0.pl
use 5.010; use strict; use warnings; use List::Util qw(sum0); say sum0( 10, 3, -8, 21 ); # 26 my @prices = (17.2, 23.6, '1.1'); say sum0(@prices); # 41.9 my @empty; say sum0(@empty); # 0
product
The product function multiplies its parameters. As this function is newer it was not constrained with backward compatibility issues so if the provided list is empty, the returned value will be 1.
examples/product.pl
use 5.010; use strict; use warnings; use List::Util qw(product); my @interest = (1.2, 2.6, 4, '1.3'); say product(@interest); # 16.224 my @empty; say product(@empty); # 1
Other functions of List::Util
The module has a number of other functions that were used in various other articles:
first
first returns the first element from a list that satisfies the given condition. For examples on how to use it an why is it good check out the articles Fast lookup by name or by date - Array - Hash - Linked List and Search for hash in an array of hashes.
any
The any function will return true if any of the given values satisfies the given condition. It is shown in the article Filtering values using Perl grep as a better solution.
It is also used in the example showing how to create a testing module and how to implement 'is_any' to test multiple expected values.
all
The all function will return true if all the supplied values satisfy the given condition. It can be seen in the article Check several regexes on many strings.
reduce
The reduce function might be familiar to you from the MapReduce programming model that was lauded around "BigData". It makes it provides a way to summarize data in an easy way. Implementing factorial in Perl - n! is a good and simple example. It is also used in the Fast lookup by name or by date - Array - Hash - Linked List article.
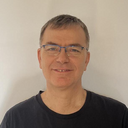
Published on 2019-04-12