exists - check if a key exists in a hash
In a hash a key-value pair have 3 different possible statuses.
The defined function checks if a value is undef or not.
The exists function check if a key is in the hash or not.
Those two conditions create 3 valid situations.
Syntax of exists
if (exists $phone_of{Foo}) { }
This code checks of the hash %phone_of has a key "Foo".
The 3 valid situations of a key-value pair
- There is a key and the value which is not undef then both the exists and defined functions will return true.
- There is a key and the value is undef then exists will return true and defined will return false.
- If there is no such key, then there cannot be a corresponding values. In that case both exists and defined will return false.
An example
examples/exists.pl
#!/usr/bin/perl use strict; use warnings; my %phones; $phones{Foo} = '111'; $phones{Qux} = undef; print exists $phones{Foo} ? "Foo exists\n" : "Foo does not exist\n"; print defined $phones{Foo} ? "Foo: defined\n" : "Foo not defined\n"; print exists $phones{Qux} ? "Qux exists\n" : "Qux does not exist\n"; print defined $phones{Qux} ? "Qux: defined\n" : "Qux not defined\n"; print exists $phones{Bar} ? "Bar exists\n" : "Bar does not exist\n"; print defined $phones{Bar} ? "Bar: defined\n" : "Bar not defined\n";
In this hash the key Foo exists and its value is defined.
The key Qux exists but its values is NOT defined.
Finally the key Bar NOT exists and if we check the defined-ness of its value we get false.
Foo exists Foo: defined Qux exists Qux not defined Bar does not exist Bar not defined
Conclusion
If defined $hash{key} is false we still don't know if the key is in the hash or not. So normally first you need to check exists $hash{key} and only then defined $hash{key}.
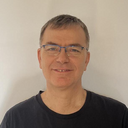
Published on 2020-08-15